; Date: Fri Jan 25 2019
Tags: Node.JS
In Node.js the require function is synchronous, meaning you're guaranteed that when require returns the module object is fully populated. But what happens when that doesn't happen? It can happen that the module object is empty, and then what do you do?
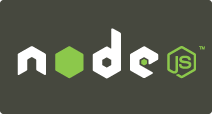
The most likely cause is a circular dependency between modules, as described here:
https://nodejs.org/api/modules.html#modules_cycles
The issue arises when module A loads module B, and then module B loads module A. That's a simple circular dependency between two modules. As is explained in the Node.js documentation:
When
main.js
loadsa.js
, thena.js
in turn loadsb.js
. At that point,b.js
tries to loada.js
. In order to prevent an infinite loop, an unfinished copy of thea.js
exports object is returned to theb.js
module.b.js
then finishes loading, and its exports object is provided to thea.js
module.
The circular dependency is detected by Node.js, and in order to avoid an infinite loop it returns the incompletely loaded module.
This is a tricky detail we can easily skip over when learning about Node.js modules. I have been writing Node.js code for almost 10 years, have written four books about Node.js programming, and had not come across this issue.
The behavior is - Your code required a module and got {}
instead.
In my case AkashaCMS, the static website generator I use to build this and other websites, suddenly started failing. After a long night of gnashing teeth and beating my head against this, all the while railing to the heavens that Node.js modules load synchronously, I finally learned about Node.js module circular dependencies.
The solution is simply to detach the circular dependencies. That's easier said than done especially since unraveling all the module circular dependencies can be difficult. The simple case presented earlier is easy to detect, but what if the dependency loop is module A uses module B which uses module C which uses module D which uses module B?
Part of your solution is Madge:
https://www.npmjs.com/package/madge
Madge is a developer tool for generating a visual graph of your module dependencies, finding circular dependencies, and give you other useful info.
Installation is simple. The authors of Madge recommend
$ npm -g install madge
You're free to do that, but as a student of the 12 Factor Application model I do not like global module installs. Therefore I installed it without the -g
option.
This is what I faced at the outset:
$ ./node_modules/.bin/madge
Usage: madge [options] <src...>
Options:
-V, --version output the version number
-b, --basedir <path> base directory for resolving paths
-s, --summary show dependency count summary
-c, --circular show circular dependencies
-d, --depends <name> show module dependents
-x, --exclude <regexp> exclude modules using RegExp
-j, --json output as JSON
-i, --image <file> write graph to file as an image
-l, --layout <name> layout engine to use for graph (dot/neato/fdp/sfdp/twopi/circo)
--orphans show modules that no one is depending on
--dot show graph using the DOT language
--extensions <list> comma separated string of valid file extensions
--require-config <file> path to RequireJS config
--webpack-config <file> path to webpack config
--ts-config <file> path to typescript config
--include-npm include shallow NPM modules
--no-color disable color in output and image
--no-spinner disable progress spinner
--stdin read predefined tree from STDIN
--warning show warnings about skipped files
--debug turn on debug output
-h, --help output usage information
MacBook-Pro-4:akasharender david$ ./node_modules/.bin/madge -c .
Processed 21 files (3s) (12 warnings)
✖ Found 10 circular dependencies!
1) filez.js > render.js > Renderer.js
2) Configuration.js > built-in.js > filez.js > render.js > index.js
3) filez.js > render.js > index.js > HTMLRenderer.js
4) index.js > HTMLRenderer.js
5) render.js > index.js > HTMLRenderer.js
6) filez.js > render.js > index.js > documents.js
7) index.js > documents.js
8) filez.js > render.js > index.js
9) render.js > index.js
10) render.js > render-json.js
There's a whole lot of options here most of which have to do with output formatting. Since I wanted to learn about circular dependencies in the AkashaRender modules, I simply used the -c
option while my shell was in the root directory of the source tree.
And -- boy did I have a lot of circular dependencies. It took an hour or so to work out how to disentangle this mess, and another hour or so to work out the follow-on problems.
How you resolve this is up to you as per your application architecture.
The goal is to achieve this state:
$ ./node_modules/.bin/madge -c .
Processed 22 files (3.4s) (12 warnings)
✔ No circular dependency found!
In my case I'd written several Classes -- Renderer
is a superclass for all Renderer objects, HTMLRenderer
is a subclass, and so forth. These classes were referenced from index.js
and several other places, and once I looked at it - yes the two files depended on each other. In index.js
the HTMLRenderer
reference was so it could export that class, and in HTMLRenderer.js
it was to utilize some AkashaRender internal functions.
The solution I decided upon was integrating what had been separate files into the same internal module. That disconnected some of the dependencies - e.g. since the Renderer class is now defined in render.js
there is no need for the two files to reference each other. The unfortunate side effect is that the internal modules are now much larger.
As was said in this
Stack Overflow posting:
Circular dependencies are code smell. If A and B are always used together they are effectively a single module, so merge them. Or find a way of breaking the dependency; maybe its a composite pattern.
Another solution mentioned in that posting is to
make sure your necessary exports are defined before you require a file with a circular dependency.
That is - since the circular dependency means that other code receives an incomplete module object, the proposed solution is to ensure the incomplete module will have the necessary objects in it.
Another
Stack Overflow posting contains some other useful hints.
Another
Stack Overflow posting makes it clear circular dependencies can arise when using ES6 Modules. And the discussion gives further useful advice on disentangling the dependencies.
This calls for a future task to re-separate these classes, and to better architect the code to not create the circular dependencies. But I'm pressed for time and uncertain how best to do that.
Bottom line
I'm surprised this problem did not come up earlier since these circular dependencies have been in place for years.
A circular dependency between Node.js modules occurs when the modules require()
each other. This dependency results in an incomplete module being loaded, and mysterious problems later.
Using Madge is an excellent way to detect the dependencies. It's up to you how you proceed from that point.