; Date: Fri Feb 22 2019
Tags: Node.JS »»»» JavaScript »»»»
Babel and Webpack are widely used tools for front-end-engineering. Between the two you can code JavaScript using the latest ES2016/2017/2018/etc features, with multiple modules, and transpile it down to a simple JavaScript file using ES5 syntax. This is a big step forward in JavaScript tooling. But, since Node.js natively supports a huge fraction of ES2016/2017/2018/etc features, why do Node.js programmers even need to know Babel/Webpack? After reading a tutorial on using Babel/Webpack with Node.js modules, I have the beginning of an inkling for why.
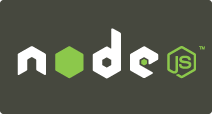
For this post we'll be referring to the following Medium post, and associated Github repository. The tutorial breezes through using Babel and Webpack to publish a Node.js module to npm. This is a very helpful tutorial if, like me, Webpack is totally mystifying.
- Tutorial:
https://medium.com/@vmarchesin/how-to-publish-a-npm-package-in-four-steps-4344ab88e852
- Repository:
git@github.com:vmarchesin/how-to-publish-a-npm-package.git
The tutorial contains a few ES6 modules that implement a couple simple functions inspired by a Python function. Because they're ES6 modules the code is written with import
and export
keywords rather than assigning functions to the module.exports
object as we do with traditional Node.js/CommonJS modules.
All that's fine, and and Node.js programmers can directly use ES6 modules using import
and export
syntax. The problem is that Node.js does not support using require
, the traditional Node.js way of consuming a module, to load an ES6 module.
To support that use-case, loading an ES6 module in a CommonJS module, look at:
https://www.npmjs.com/package/esm -- While that module does the job it is not fair to the consumers of your Node.js module to require them to use a third-party module to then load your module.
Three rationales:
- Suppose you want to code using ES6 module syntax, but want to use the resulting module in a CommonJS module? Node.js does not support
require('path/to/module.mjs')
, meaning that a CommonJS module cannot load an ES6 module usingrequire
. - Suppose you totally despise the
.mjs
file extension required to use ES6 modules with Node.js. Babel can be used to rewrite.js
files containing an ES6 module into a.js
file containing a CommonJS module. - Suppose your management requires using an older Node.js release that lacks ES6 module support? You can write code with all the latest goodness, then use Babel to transpile the code to ES5 syntax until your management gets a clue.
Between those three, we have sufficient rationale to use Babel for publishing a Node.js module to the npm repository.
But... why use Webpack? I'm still a little mystified. The only rationale I can come up with is obfuscation. To see why let's go through the tutorial and the results.
This helped me a lot to have a beginning of an inkling how Webpack is used. You can follow this and see the entrypoint to the module is src/index.js
, that Webpack is to package anything referred from that file, the output is put into dist/index.js
which will be written in CommonJS format.
const path = require('path');
module.exports = {
mode: 'production',
entry: './src/index.js',
output: {
path: path.resolve('dist'),
filename: 'index.js',
libraryTarget: 'commonjs2',
},
module: {
rules: [
{
test: /\.js?$/,
exclude: /(node_modules)/,
use: 'babel-loader',
},
],
},
resolve: {
extensions: ['.js'],
},
};
Then, the result of that is:
module.exports=function(e){var t={};function r(n){if(t[n])return t[n].exports;var o=t[n]={i:n,l:!1,exports:{}};return e[n].call(o.exports,o,o.exports,r),o.l=!0,o.exports}return r.m=e,r.c=t,r.d=function(e,t,n){r.o(e,t)||Object.defineProperty(e,t,{enumerable:!0,get:n})},r.r=function(e){"undefined"!=typeof Symbol&&Symbol.toStringTag&&Object.defineProperty(e,Symbol.toStringTag,{value:"Module"}),Object.defineProperty(e,"__esModule",{value:!0})},r.t=function(e,t){if(1&t&&(e=r(e)),8&t)return e;if(4&t&&"object"==typeof e&&e&&e.__esModule)return e;var n=Object.create(null);if(r.r(n),Object.defineProperty(n,"default",{enumerable:!0,value:e}),2&t&&"string"!=typeof e)for(var o in e)r.d(n,o,function(t){return e[t]}.bind(null,o));return n},r.n=function(e){var t=e&&e.__esModule?function(){return e.default}:function(){return e};return r.d(t,"a",t),t},r.o=function(e,t){return Object.prototype.hasOwnProperty.call(e,t)},r.p="",r(r.s=0)}([function(e,t,r){"use strict";r.r(t);var n=(e,t)=>Boolean(Math.round(Math.random()));var o=(e,t)=>Math.random()*(e-t)+t;var u=(e,t)=>~~(Math.random()*(e-t+1)+t);t.default=((e=1,t=0,r={})=>!0===r.float?o(e,t):r.bool?n(e,t):u(e,t))}]);
I dunno about you, but I have a hard time following this. I even manually cleaned this up hoping that would help, but the cleaned up code was still hard to follow.
If you want to obfuscate the module for whatever reason, this is a great way to go. Me? I don't care much about obfuscating the Node.js modules I publish to npm.
Another possible rationale for using Webpack is its guarantee of minimizing the code. You can see some code minimization here, with all the single-letter variable and function names. But Webpack does even more by leaving out any unreferenced code as it packages your code.
This blob shown here is the result of smushing together four separate modules. During the smushing process, if Webpack were to find a function that's not referenced from anywhere it'll simply leave out that function. Therefore your module has two execution-time advantages: a) faster parsing time because the code is all scrunched up, b) minimized memory footprint because it's all one module and because unneeded code is left out.