Tags: Node.JS »»»» Asynchronous Programming
With today's first release of Node.js version 7, we now have async/await as a base feature of the platform. This is a significant milestone that wasn't even mentioned in the commit message. The async/await feature is so important that I'm preparing a short book to discuss JavaScript asynchronous programming patterns, that highly features async/await.
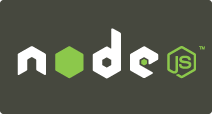
$ nvm install 7
Downloading https://nodejs.org/dist/v7.0.0/node-v7.0.0-darwin-x64.tar.xz...
######################################################################## 100.0%
That's how easy it is now to install Node.js version 7, if you have nvm installed. Implementing async/await is just a command line option away.
'use strict';
const fs = require('fs-extra-promise');
const util = require('util');
async function twofiles() {
var texts = [
await fs.readFileAsync('hello.txt', 'utf8'),
await fs.readFileAsync('goodbye.txt', 'utf8')
];
console.log(util.inspect(texts));
}
twofiles();
Try this code ... Run npm init
to generate a package.json
, and then: npm install fs-extra-promise --save
Finally, put suitable text into hello.txt
and goodbye.txt
...
Your first attempt at running this might result in this:
$ node 2files.js
/Users/david/ebooks/Callbacks-Promises-Async/examples/asyncawait/2files.js:6
async function twofiles() {
^^^^^^^^
SyntaxError: Unexpected token function
at Object.exports.runInThisContext (vm.js:76:16)
at Module._compile (module.js:545:28)
at Object.Module._extensions..js (module.js:582:10)
at Module.load (module.js:490:32)
at tryModuleLoad (module.js:449:12)
at Function.Module._load (module.js:441:3)
at Module.runMain (module.js:607:10)
at run (bootstrap_node.js:382:7)
at startup (bootstrap_node.js:137:9)
at bootstrap_node.js:497:3
That's because you're missing a flag. The async/await feature is behind a --harmony
flag. Run this instead:
$ node --harmony-async-await 2files.js
[ 'Hello, world!\n', 'Goodbye, world!\n' ]
Have fun. Ponder the code above. It is so fetchingly simple, yet does asynchronous execution. This is our future. I'll shortly have an initial version of my book available.