; Date: Fri Jun 08 2018
Tags: Amazon Web Services »»»» AWS DynamoDB »»»»
The AWS SDK for JavaScript/Node.js documentation describes receiving results the old way, using a callback function. In the modern era of JavaScript we know what to do -- we wrap such functions in await new Promse, hold our nose, and embed the callback within the Promise. I published an article on the Sourcerer blog describing how to interact with DynamoDB from a Node.js Lambda function, and then a reader asked why it used "await new Promise". A bit of searching revealed that indeed Amazon had updated the SDK to support Promises, but it seems the SDK documentation has not been updated. What follows is a quick example of an AWS Lambda function calling DynamoDB methods using Promise objects in an async/await, and deployed using the Serverless Framework.
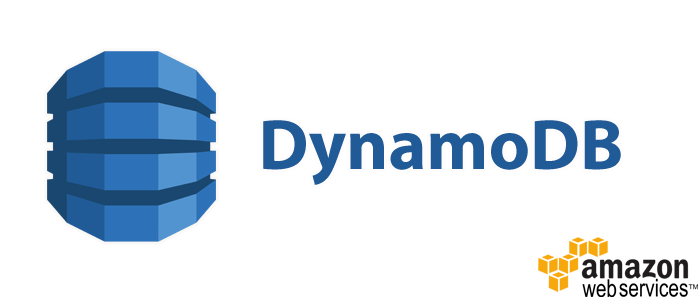
With the Serverless Framework it's possible to quickly implement Node.js Lambda functions using any AWS service. For a simple example, see Getting started with using Node.js and the Serverless framework on AWS Lambda
In this case at 5:30 PM I received email notification that a reader had replied to the newly posted article on the Sourcerer blog saying:
Why
await new Promise
? Why not just query DynamoDB using promises in the first place instead of callbacks? Good article, but the example snippets could have been better.
By 7:40 PM I'd read the response, had an initial response ("because the SDK doesn't support that"), then thought it would be better to see if it does support it, found the announcement that the AWS SDK for Node.js does support Promise's, digested in my mind how to implement the support in my application, updated the application for the article, tested that implementation, updated the article, then read a few posts on Quora, watched a could YouTube videos, then thought I might write this blog post, wrote a quick sample application, and debugged that application.
In other words - in about 2 hours I did rewrote one application, and then wrote a whole new Serverless Framework application. And, by 8:30 PM this blog post was written. Plus did some other futzing around doing unrelated stuff on Facebook/Quora/YouTube. That's pretty impressive, but it is also a tangent from what we're here to do.
Namely -- how to get a Promise out of AWS SDK for JavaScript method calls.
Supposedly, AWS SDK for JavaScript does not support Promises
There are three places this SDK is documented:
-
docs.aws.amazon.com sdk-for-javascript v2 developer-guide/welcome.html
-
docs.aws.amazon.com AWSJavaScriptSDK latest AWS DynamoDB.html
-
docs.aws.amazon.com AWSJavaScriptSDK latest AWS DynamoDB DocumentClient.html
So far as I could find, none of them discuss how to get Promise objects out of an SDK call.
Since we all should be doing our best to use async/await functions, and to use functions that return Promise objects, having an SDK lacking support for Promises is inconvenient. But there is a simple workaround - wrap the function inside an await new Promise
construct.
In other words,
return await new Promise((resolve, reject) => {
dynamoDb.put(params, (error, data) => {
if (error) {
console.log(`createChatMessage ERROR=${error.stack}`);
resolve({
statusCode: 400,
error: `Could not create message: ${error.stack}`
});
} else {
console.log(`createChatMessage data=${JSON.stringify(data)}`);
resolve({ statusCode: 200, body: JSON.stringify(params.Item) });
}
});
});
That code comes directly from
the article I posted on the Sourcerer blog. The pattern is -
- Create a Promise (
new Promise
) - In the Promise callback invoke your asynchronous function
- In the callback for that asynchronous function use
resolve
andreject
to show results to the Promise object - By using
await new Promise
on this, theresolve
andreject
states will be reflected either in a thrown error, or data
This pattern is suboptimal, but there is a lot of older code that does not return Promises and therefore we have to know this workaround.
Getting Promise objects from AWS SDK method calls
Announcement:
aws.amazon.com blogs developer support-for-promises-in-the-sdk
It says:
By default, the AWS SDK for JavaScript will check for a globally defined Promise function. If found, it adds the promise() method on AWS.Request objects. Some environments, such as Internet Explorer or earlier versions of Node.js, don’t support promises natively. You can use the AWS.config.setPromisesDependency() method to supply a Promise constructor. After this method is called, promise() will exist on all AWS.Request objects. The following example shows how you can set a custom promise implementation for the AWS SDK to use.
When reading that announcement ignore all the stuff about setting up a Promise library. At that time Node.js did not have native Promise support, and 3rd party libraries were required instead. Instead, focus on this:
- When invoking an AWS SDK function, leave out the callback function in the documentation.
- Leaving out the callback function causes it to return an
AWS.Request
object - The
AWS.Request
object has apromise
method that starts the requested operation, and returns a Promise - That Promise can be used in an async/await function.
For example:
const data = await dynamoDb.query(params).promise();
Read on to see precisely what to do.
Demonstrating AWS SDK Promises with a tiny Serverless application
Elsewhere I demonstrate how to setup the Serverless framework on your laptop: Getting started with using Node.js and the Serverless framework on AWS Lambda
Run: serverless create --template aws-nodejs
to setup a blank application.
Edit the serverless.yml
to be:
service: aws-nodejs-promise
custom:
tableName: 'aws-nodejs-promise-table-${self:provider.stage}'
provider:
name: aws
runtime: nodejs8.10
stage: dev
region: us-east-1
environment:
MESSAGES_TABLE: ${self:custom.tableName}
AWS_DEPLOY_REGION: ${self:provider.region}
iamRoleStatements:
- Effect: Allow
Action:
- dynamodb:Query
- dynamodb:Scan
- dynamodb:GetItem
- dynamodb:PutItem
- dynamodb:UpdateItem
- dynamodb:DeleteItem
Resource:
- { "Fn::GetAtt": ["PostFetchDynamoDBTable", "Arn" ] }
functions:
post:
handler: handler.post
events:
- http:
method: POST
path: /post
private: false
fetch:
handler: handler.fetch
events:
- http:
method: GET
path: /fetch
private: false
resources:
Resources:
PostFetchDynamoDBTable:
Type: AWS::DynamoDB::Table
Properties:
AttributeDefinitions:
- AttributeName: messageId
AttributeType: S
KeySchema:
- AttributeName: messageId
KeyType: HASH
ProvisionedThroughput:
ReadCapacityUnits: 1
WriteCapacityUnits: 1
TableName: ${self:custom.tableName}
This sets up a simple HTTP service along with a simple DynamoDB table along with the permissions required to use the table.
A POST on /post
will cause data to be pushed into the table, and a GET on /fetch
will retrieve data. We're going for concise demonstration rather than a comprehensively correct application.
Edit handler.js
to be:
const AWS = require('aws-sdk');
const MESSAGES_TABLE = process.env.MESSAGES_TABLE;
const AWS_DEPLOY_REGION = process.env.AWS_DEPLOY_REGION;
const dynamoDb = new AWS.DynamoDB.DocumentClient({
api_version: '2012-08-10',
region: AWS_DEPLOY_REGION
});
module.exports.post = async (event, context) => {
const params = {
TableName: MESSAGES_TABLE,
Item: {
messageId: `${Math.random()}`,
message: "Hello World!"
},
};
try {
const data = await dynamoDb.put(params).promise();
return { statusCode: 200, body: JSON.stringify({ params, data }) };
} catch (error) {
return {
statusCode: 400,
error: `Could not post: ${error.stack}`
};
}
};
module.exports.fetch = async (event, context) => {
const params = {
TableName: MESSAGES_TABLE
};
try {
const data = await dynamoDb.scan(params).promise();
return { statusCode: 200, body: JSON.stringify(data) };
} catch (error) {
return {
statusCode: 400,
error: `Could not fetch: ${error.stack}`
};
}
};
The data that's posted is simply a random number and the text Hello World. The data is pushed to the DynamoDB table with a put
operation. The /fetch
operation uses scan
to read the entire table.
The important thing here is the pattern:
try {
const data = await dynamoDb.scan(params).promise();
return { statusCode: 200, body: JSON.stringify(data) };
} catch (error) {
return {
statusCode: 400,
error: `Could not fetch: ${error.stack}`
};
}
The success side of the try/catch
performs the query, and if it does not fail the result is returned. Otherwise an error indicator is returned. The .promise()
thing is what makes it work as a Promise.
Indeed, a POST to https://OBSCURED.execute-api.us-east-1.amazonaws.com/dev/post
results in:
{
"params": {
"TableName": "aws-nodejs-promise-table-dev",
"Item": {
"messageId": "0.4285571055760491",
"message": "Hello World!"
}
},
"data": {}
}
And a GET on https://OBSCURED.execute-api.us-east-1.amazonaws.com/dev/fetch
results in:
{
"Items": [
{
"messageId": "0.6731153787359891",
"message": "Hello World!"
}
],
"Count": 1,
"ScannedCount": 1
}