; Date: Sat Jun 09 2018
Recaptcha has been around a long time, fighting the good fight against spammers. One of my sites has been using Recaptcha for years, long enough to require updating to new API implementations a couple times. Google has updated to Recaptcha v2 and soon v3, and somehow my site stopped enforcing Recaptcha's with the subsequent Spammer intrusions. Fixing the implementation proved to be difficult, especially as the available tutorials missed some details.
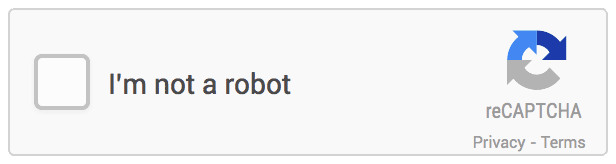
Near as I can recall, when I started using Recaptcha it was not owned/operated by Google. After awhile, Google took over the service. Initially the sales pitch was "Fight Spam, Read Books" because the Recaptcha images used in that time period were image excerpts from scanned books. The Recaptcha service was used to crowdsource validation of algorithms to read text out of scanned images.
After Google took over, the service switched over to verifying data gathered from Google's Street View cars. In other words, Google needed us all to verify text in street signs and house number signs.
Nowadays the Recaptcha service now gives us a simple checkbox, and Google's code does some kind of heuristics to rationalize whether it's a human or not. If Google's code becomes suspicious only then will it start presenting challenges, such as images captured from Google Street View cars.
The basic idea of Recaptcha is two steps ...
- In a page containing a FORM, add a Recaptcha widget where the user is presented with something that tries to determine whether it is a human
- In the code that receives the FORM, query the Recaptcha service to see if the user proved themselves.
The problem discovered today is that Recaptcha's stopped working on one of my websites, and spammers were sending their bogons to that website. Recaptcha has been working on that site for years. It's not clear why it would have begun to fail.
While debugging I found that the Recaptcha verification token did not arrive on the receiving side. It took awhile to work out why that happened - so read on for the solution.
Google's Recaptcha home page:
https://developers.google.com/recaptcha/
A first step to adopting Recaptcha on your site is to get an API key. See:
http://www.google.com/recaptcha/admin
During the process of getting an API key, you'll be given a pair of keys - one of which is SECRET.
For a page with an HTML FORM to protect, you'll be told to put this in the header:
<script src="https://www.google.com/recaptcha/api.js" async defer></script>
And then to put this inside the FORM
<div class="g-recaptcha" data-sitekey="CLIENT KEY"></div>
The JavaScript then munges any div.g-recaptcha
injecting code for the Recaptcha form. Within that code is a textarea.g-recaptcha-response
field. When the FORM is submitted the contents of that field is submitted as well. Google's JavaScript -- if it determines the user is safe -- will insert a token into that field, and therefore the token will be received by the code which recieves the FORM data.
See the documentation for more details:
https://developers.google.com/recaptcha/docs/display
The user has submitted the form - and the submitted data includes information from the .g-recaptcha-response
field. How does your code determine whether it was okay?
- For a
GET
FORM, the FORM values, including the.g-recaptcha-response
field, will be added to the URL as query parameters - For a
POST
FORM, the FORM values, including the.g-recaptcha-response
field, will be in the request body
Many of the tutorials assume a POST
form and therefore demonstrate accessing values from the request body.
The code receiving the request needs to parse both - and most app frameworks will make these values available in a request object. Your code cannot just look at that form value and assume all is well if that field is non-empty. A miscreant could fill that field with bogus data, and your code wouldn't be any the wiser.
Instead what your code must do is make a verification request at https://www.google.com/recaptcha/api/siteverify
with parameters which includes your SECRET API KEY and the .g-recaptcha-response
field. That value is generated on the originating page by Google Recaptcha client code. The verification request is meant to validate the .g-recaptcha-response
field is holding trustable information. Your code is to use the returned object to validate this user.
The method to do that request varies from language to language. For discussion sake let's look at an implementation in PHP.
function CaptchaVerify()
{
$apiSecret = "SECRET KEY";
// From: https://www.kaplankomputing.com/blog/tutorials/recaptcha-php-demo-tutorial/
$response;
if (isset($_POST['g-recaptcha-response']) && !empty($_POST['g-recaptcha-response'])) {
$response = $_POST['g-recaptcha-response'];
} else if (isset($_GET['g-recaptcha-response']) && !empty($_GET['g-recaptcha-response'])) {
$response = $_GET['g-recaptcha-response'];
}
if (isset($response) && !empty($response)) {
$url = 'https://www.google.com/recaptcha/api/siteverify';
$data = [
'secret' => $apiSecret,
'response' => $response
];
$options = [
'http' => [
'method' => 'POST',
'content' => http_build_query($data)
]
];
$context = stream_context_create($options);
$verify = file_get_contents($url, false, $context);
$captcha_success = json_decode($verify);
if ($captcha_success->success == false) {
return false;
} else {
return true;
}
} else {
return false;
}
/*
if (isset($_POST['g-recaptcha-response']) && !empty($_POST['g-recaptcha-response'])) {
// From: https://stackoverflow.com/questions/27274157/new-google-recaptcha-with-checkbox-server-side-php
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, "https://www.google.com/recaptcha/api/siteverify");
curl_setopt($ch, CURLOPT_HEADER, 0);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS, [
'secret' => $apiSecret,
'response' => $_POST['g-recaptcha-response'],
'remoteip' => $_SERVER['REMOTE_ADDR']
]);
$resp = json_decode(curl_exec($ch));
curl_close($ch);
if ($resp->success) {
return true; // Success
} else {
return false; // failure
}
} else {
echo "Recaptcha not supplied";
return false;
}
*/
// Comes from: https://stackoverflow.com/questions/27264459/struggling-with-recaptcha-v2-and-form-submission
//Returns -1 if no captcha response was in post(user did not submit form)
//Returns 0 if the verification fails
//Returns 1 if the verification succeeds
/* $captcha = isset($_POST['g-recaptcha-response'])? "&response=".$_POST['g-recaptcha-response']:'';
if($captcha != '')
{
$verifyUrl = "https://www.google.com/recaptcha/api/siteverify";
$apiSecret = "?secret=".$apiSecret;
$remoteip = "&remoteip=".$_SERVER['REMOTE_ADDR'];
$response=file_get_contents($verifyUrl.$apiSecret.$captcha.$remoteip);
$obj = json_decode($response);
return (integer)$obj->success;
}
return -1; */
}
There are three different implementations because I found several alternative tutorials. The one that's uncommented was the best, however the tutorial where this was found was not sufficient.
That tutorial assumed the form values would arrive in PHP as entries in the $_POST
array. That is only true for a POST-style FORM. In my case I had a GET-style FORM, and the form values instead arrived in the $_GET
array.
It took a long enough time to figure that out, that I felt it was worthwhile documenting this. You'll see that my code first checks the $_POST
array, then the $_GET
array.