; Date: Thu Aug 02 2018
Web applications obviously will need CSS, and therefore Webpack must support packaging applications with CSS files. However with the sample Webpack build procedure I'm using, it fails while loading a CSS file. Let us take a look at the configuration changes required to fix this problem.
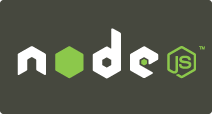
I'm developing a Vue.js component that I hope to deliver through the npm repository. I've already solved one problem having to do with loading JSON modules with Webpack. With that problem out of the way, I've come across another problem:
ERROR in ./node_modules/sl-vue-tree/dist/sl-vue-tree-dark.css 1:0
Module parse failed: Unexpected token (1:0)
You may need an appropriate loader to handle this file type.
> .sl-vue-tree {
| position: relative;
| cursor: default;
@ ./src/vue-file-tree.vue (./node_modules/vue-loader/lib/selector.js?type=script&index=0!./src/vue-file-tree.vue) 66:0-47
@ ./src/vue-file-tree.vue
Obviously it is unable to load CSS files. Looking in the Webpack for another package I see this code:
const ExtractTextPlugin = require('extract-text-webpack-plugin');
...
module.exports = {
...
module: {
rules: [
{
test: /\.css$/,
use: ExtractTextPlugin.extract({
fallback: 'style-loader',
use: 'css-loader'
})
},
{
test: /\.html$/,
use: 'vue-html-loader'
},
...
]
...
}
...
};
BUT -- IGNORE THIS BECAUSE IT IS INCOMPATIBLE WITH WEBPACK v4 ... SEE BELOW INSTEAD FOR USING mini-css-extract-plugin
After running this:
IGNORE THIS $ npm install extract-text-webpack-plugin --save
IGNORE THIS AS WELL I get the following error:
$ npm run build
...
ERROR in ./src/vue-file-tree.vue (./node_modules/vue-loader/lib/selector.js?type=script&index=0!./src/vue-file-tree.vue)
Module not found: Error: Can't resolve 'style-loader' in '/Volumes/Extra/akasha-tools/vue-file-tree'
@ ./src/vue-file-tree.vue (./node_modules/vue-loader/lib/selector.js?type=script&index=0!./src/vue-file-tree.vue) 66:0-47
@ ./src/vue-file-tree.vue
...
That's a step forward but still an error.
That's fixed with:
IGNORE THIS $ npm install style-loader --save
But another error crops up:
ERROR in ./node_modules/sl-vue-tree/dist/sl-vue-tree-dark.css
Module build failed (from ./node_modules/extract-text-webpack-plugin/dist/loader.js):
Error: "extract-text-webpack-plugin" loader is used without the corresponding plugin, refer to https://github.com/webpack/extract-text-webpack-plugin for the usage example
at Object.pitch (/Volumes/Extra/akasha-tools/vue-file-tree/node_modules/extract-text-webpack-plugin/dist/loader.js:57:11)
@ ./src/vue-file-tree.vue (./node_modules/vue-loader/lib/selector.js?type=script&index=0!./src/vue-file-tree.vue) 66:0-47
@ ./src/vue-file-tree.vue
Which has to do with having been required to add this to the plugins
array:
IGNORE THIS new ExtractTextPlugin("styles.css"),
Now the error is:
Error: Chunk.entrypoints: Use Chunks.groupsIterable and filter by instanceof Entrypoint instead
at Chunk.get (/Volumes/Extra/akasha-tools/vue-file-tree/node_modules/webpack/lib/Chunk.js:824:9)
at /Volumes/Extra/akasha-tools/vue-file-tree/node_modules/extract-text-webpack-plugin/dist/index.js:176:48
at Array.forEach (<anonymous>)
at /Volumes/Extra/akasha-tools/vue-file-tree/node_modules/extract-text-webpack-plugin/dist/index.js:171:18
at AsyncSeriesHook.eval [as callAsync] (eval at create (/Volumes/Extra/akasha-tools/vue-file-tree/node_modules/tapable/lib/HookCodeFactory.js:24:12), <anonymous>:12:1)
at AsyncSeriesHook.lazyCompileHook [as _callAsync] (/Volumes/Extra/akasha-tools/vue-file-tree/node_modules/tapable/lib/Hook.js:35:21)
at Compilation.seal (/Volumes/Extra/akasha-tools/vue-file-tree/node_modules/webpack/lib/Compilation.js:1203:27)
at hooks.make.callAsync.err (/Volumes/Extra/akasha-tools/vue-file-tree/node_modules/webpack/lib/Compiler.js:547:17)
at _err0 (eval at create (/Volumes/Extra/akasha-tools/vue-file-tree/node_modules/tapable/lib/HookCodeFactory.js:24:12), <anonymous>:11:1)
at _addModuleChain (/Volumes/Extra/akasha-tools/vue-file-tree/node_modules/webpack/lib/Compilation.js:1054:12)
at processModuleDependencies.err (/Volumes/Extra/akasha-tools/vue-file-tree/node_modules/webpack/lib/Compilation.js:980:9)
at process._tickCallback (internal/process/next_tick.js:61:11)
The correct solution -- SKIP DOWN TO HERE
This error arises because up at the start I'd chosen the wrong CSS processor. I've left the incorrect advice in place above to show some of the incorrect results.
https://stackoverflow.com/questions/51383618/chunk-entrypoints-use-chunks-groupsiterable-and-filter-by-instanceof-entrypoint
Since webpack v4 the extract-text-webpack-plugin should not be used for css. Use mini-css-extract-plugin instead.
This plugin has instructions at:
https://github.com/webpack-contrib/mini-css-extract-plugin
In the Webpack config use this instead:
const MiniCssExtractPlugin = require("mini-css-extract-plugin");
...
module.exports = {
...
module: {
rules: [
{
test: /\.css$/,
use: [
{
loader: MiniCssExtractPlugin.loader,
options: {
// you can specify a publicPath here
// by default it use publicPath in webpackOptions.output
publicPath: '../'
}
},
"css-loader"
]
},
...
]
...
}
...
plugins: [
...
new MiniCssExtractPlugin({
// Options similar to the same options in webpackOptions.output
// both options are optional
filename: "[name].css",
chunkFilename: "[id].css"
})
]
...
}
...
Once you have this plugin installed, run: $ npm run build
The build should work correctly at that point.