; Date: Wed Jul 02 2014
Tags: Node.JS
Node.js is horrible with CPU bound processing, supposedly. Why? Because CPU-intensive algorithms block the event loop from handling events, blocking the Node.js platform from doing its core competency. Actually, as I demonstrate in my book Node Web Development (see sidebar for link), it's possible to use "setImmediate" to dispatch work through the Node.js event loop, and perform intensive computation while not blocking the event loop. The example I chose for the book is a simplistic Fibonacci algorithm, and requesting "large" Fibonacci values (like "50") would take a loooong time. But, by recoding the Fibonacci algorithm using setImmediate, it can do calculation for any Fibonacci value without blocking the event loop.
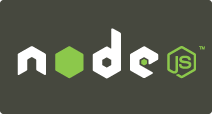
However, this isn't always what you want to do. The CPU-intensive processing is still running in the Node.js process, it's just not blocking the event loop. That's an improvement, but not always what we want.
In particular it should be good to move any CPU-intensive stuff out of the user-facing server. That way the outside edge of your application will remain as responsive as possible.
With the Fibonacci algorithm there's a fairly obvious move, and that's to not use such a simplistic Fibonacci algorithm. For example, caching Fibonacci values is quite easy, and will result in nearly instantaneous Fibonacci calculations of any value. But, calculating Fibonacci values is not an example of real world CPU-intensive work. Maybe you can make drastic performance improvements in your algorithm, but probably not.
What you can do is farm CPU-intensive work out to other servers. The question is - how?
A really simple way, and one that I show in Node Web Development, is that Express makes it trivially easy to create a REST server. You may think of Express as being a framework for HTML webapp development, but it's also useful for REST services.
It's as simple as building a server with an Express route definition like this:
app.get('/fibonacci/:n', function(req, res, next) {
calculateCPUintensiveValue(req.params.n, function(err, result) {
if (err) next('ERROR OCCURRED');
else {
res.send({ n: req.params.n, result: result });
}
});
});
Of course you should use a pathname appropriate to your application, and your application is probably going to expose more API points. This is the pattern, though, of putting request parameters in the URL, and when done with the CPU-intensive calculation you call res.send. That function formats the object it's given as JSON, automatically.
Once you've coded your server, it can be tested easily:
$ curl -f http://localhost:3000/fibonacci/10
{ "n": "10", "result": 55 }
And then to call the REST service from inside another Express application, do this:
var httpreq = require('http').request({
host: "localhost", port: 3000,
path: "/fibonacci/"+ Math.floor(fiboRequest),
method: "GET"
}.function(httpresp) {
httpresp.on('data', function(chunk) {
var data = JSON.parse(chunk);
res.render(... );
});
httpreq.end();
};
The simplicity of doing this is one of the big wins from using Node.js. The platform and the major libraries, like Express, work together to make this sort of task easy as pie.