Tags: Node.JS
Neither Node.js nor ExpressJS have an opinion about the best folder structure. As a non-opinionated application framework, Express doesn't much care how you wish to organize your code. Since the folder organization is up to you, please consider the following as one among many structures that at least one programmer thinks is an efficient way to organize Express application code. The structure here more-or-less follows the Model-View-Controller paradigm, meaning we have separated the code for data models and data persistence from the business logic from the view code.
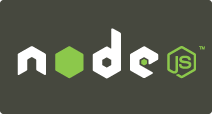
We're talking about the folder structure for a Node.js app written using Express. There are two existing constraints on the folder structure:
- The
node_modules
is baked-in-to Node.js, and is where the package dependencies live. You can't do anything about this directory, since Node.js requires its existence. You create apackage.json
listing the dependencies, then runnpm install
to install the dependencies andnpm update
to update them. - The
views
directory is where the templates live -- set this up withapp.set('views', './views')
and notice you can use any directory name you like - The
static
directory contains static web content like browser-side JavaScript and CSS files, images, fonts, smellovision, etc -- Configure usingapp.use(express.static('static'))
and notice you can use any directory name you like
In your views
directory are template files with file-name extensions matching the view engine being used. If using Handlebars, the configuration is app.set('view engine', 'hbs')
and the template files are named like index.hbs
Some template engines support partials, which are snippets of template code that can be reused. Therefore one needs a directory for these files. Our folder structure is starting to flesh out to:
node_modules
views
static
partials
There must be a top-level file that wires together the application. This file should have minimal logic, and instead focus on the declarations necessary to set up the structure. If you use express-generator
you see an app.js
that is pretty much right-on, and another file bin/www
that's meant to launch a stand-alone version of the application.
node_modules
views
static
partials
app.js
bin/www
So far we've covered the View portion of the Model-View-Controller paradigm.
In Express, Router functions are the closest analogue to the Controller part of the MVC paradigm. Router functions include both Middleware functions as well as the Routing functions. Middleware functions are useful across many routes - an example are the cookie-parser
and body-parser
modules, and you can use Middleware to implement access control to each URL.
Middleware could be implemented as separate modules that are loaded as an external dependency using package.json
. Or you could have middleware just for this application. In the former, the middleware modules are in node_modules
, while for the latter you might have a directory for them.
The Router functions should be in their own directory, routes
. Express-generator sets up a routes
directory, FWIW.
node_modules
views
static
partials
app.js
bin/www
routes
middleware
The last part of the MVC model is the Model. Technically speaking the Model is the data structure handed to the View for display to the user. But, what about the code that generates the Model data structure, and what about database persistence of the application data?
Also, with care one can implement the same Model API on top of multiple persistence engines. In the Node.js universe there are many choices for persisting data, from low-level database drivers, to ORM libraries like Sequalize (for SQL databases) and MongooseJS (for MongoDB).
node_modules
views
static
partials
app.js
bin/www
routes
middleware
models
models-sequelize
(optional)models-mongoose
(optional)models-xyzzy
(if exploring Collosal Cave)
The last three are examples of side-by-side implementations of a Model API on top of different database layers.