; Date: Sat Jul 21 2018
Tags: Vue.js »»»» JavaScript
As a follow-up to Add/remove list items in Vue.js built with Bulma/Buefy we want to study form validation. That example includes a little form in which we enter some information, and we want a little bit of validation to make sure we don't add bad entries.
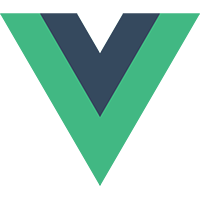
If you haven't already done so, go over the companion tutorial: Add/remove list items in Vue.js built with Bulma/Buefy
You'll need that code to run this tutorial.
Change the <template>
in App.vue
to this:
<template>
<div id="list-add-delete" class="columns">
<section id="items-area" class="column">
<ul id="item-list">
<li v-for="(item, index) of items" v-bind:key="index" :id="item.id">
{{ item.item }}
<button class="button is-medium is-primary"
@click="removeItem(index)"><b-icon icon="close-box-outline"></b-icon></button>
</li>
</ul>
</section>
<section id="new-item-form" class="column">
<div class="field">
<b-switch v-model="deleteWithSplice">
{{ deleteWithSplice ? ".splice" : "$delete" }}
</b-switch>
</div>
<b-field horizontal label="id" :type="isIDlabelDanger" :message="IDLabelMessage">
<b-input v-model="newId"></b-input>
</b-field>
<b-field horizontal label="label" :type="isItemLabelDanger" :message="ItemLabelMessage">
<b-input v-model="newItem"></b-input>
</b-field>
<button class="button is-medium is-primary"
:disabled="isNewItemFormDisabled"
@click="addNewItem">Add</button>
</section>
</div>
</template>
What we've done is add a few classes to the <b-field>
elements. In the Bulma/Buefy documentation the type
and message
attributes can be used as indicators that something is wrong in the input field. The type
attribute has several possible values and we'll use is-danger
to represent something is wrong. The message
attribute will be used to display an explanation to the user.
A typical approach is for form elements with insane values, to draw a red border around the element, and to put a message below the element. Buefy/Bulma gives us that capability, when is-danger
is set, and the type
and message
attributes are the first step.
For the next step, add this section in the data exported from <script>
element
computed: {
isIDlabelDanger() {
if (this.newId === "") return "is-danger";
let hasItem = this.hasItemById(this.newId);
if (hasItem) {
return "is-danger";
} else {
return "is-success";
}
},
IDLabelMessage() {
if (this.newId === "") return "Please enter an ID";
let hasItem = this.hasItemById(this.newId);
if (hasItem) {
return "Please enter a unique ID";
} else {
return "";
}
},
isItemLabelDanger() {
if (this.newItem !== "") {
return "is-success";
} else {
return "is-danger";
}
},
ItemLabelMessage() {
if (this.newItem !== "") {
return "";
} else {
return "Please enter an Item";
}
},
formIsInDanger() {
if (this.isIDlabelDanger === "is-danger"
|| this.isItemLabelDanger === "is-danger") {
return true;
} else {
return false;
}
},
isNewItemFormDisabled() {
if (this.formIsInDanger) return true;
else return false;
}
},
In Vue.js the computed
object contains functions that, as the name implies, help with computing values. See
https://vuejs.org/v2/api/#computed
These are similar to methods but cache the result like other properties. This means computed methods are not reinvoked unless needed, whereas methods are.
In this case the computed properties are associated with the type
and message
attributes, and compute the value for those attributes based on data stored by the application.
It boils down to setting either is-danger
or is-success
depending on whether the values are okay. This is computed using the formIsInDanger
function. Using Vue.js syntax (:type="isIDlabelDanger" :message="IDLabelMessage"
) these component values are dynamically bound to the component so that the component is automatically updated as the user works with the application.
Another property is attached to the Add
button. We want to prevent the user from even clicking that button unless the form elements are acceptable. Using :disabled="isNewItemFormDisabled"
this button is either disabled, or not, depending on whether the form elements are in is-danger
, or not.
The code depends on a function, hasItemById
which must be added to the methods object:
methods: {
...
hasItemById(id) {
let hasItem = false;
if (id === "") return hasItem;
for (let item of this.items) {
if (item.id === id) {
hasItem = true;
break;
}
}
return hasItem;
}
}
This function looks through the items
array to see if one already has the given id
value. The purpose here is to enforce the rule that there must be only one HTML element on a given page with the given id
value. The function detects for us whether a proposed id
is already in use.
What we've done is various forms of feedback showing whether form values are acceptable - and then disabling the form if form values are not acceptable.
It's simple and straightforward to implement and is somewhat effective. What was promised at the top is simple form validation not comprehensive form validation. Obviously there are other things which fall in the camp of form validation, so this is only the start.