; Date: Fri Jan 05 2018
Tags: Spring Boot
You're coding a Spring Boot application, need a database, and quickly configure in the H2 in-memory database thinking it'll be easy. Rerun the application, do a few things, then try to get into the H2 console, and it's a blank screen. The JavaScript console says "Refused to display ... in a frame because it set 'X-Frame-Options' to 'deny'." and there's a sinking feeling, it's another one of those obtuse error messages from the bowels of somewhere you've never heard of. Fortunately the solution is easy.
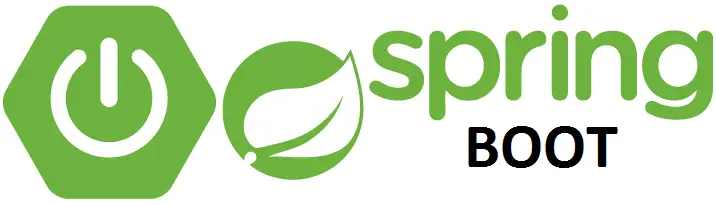
In my case I'm playing with the Spring Social Showcase demo because I need to add OAuth2 support to the application I'm writing. The Spring Social project is supposed to make this all easy as pie.
There is a database table that's generated by the Showcase demo, and I wanted to view the database. In my application.yml
I'd configured the H2 database as so:
spring
datasource:
name: orangebutton
jdbc-url: jdbc:h2:mem:orangebutton
platform: h2
h2:
console:
enabled: true
path: /console
Therefore visiting http://localhost:8080/console
and logging into the H2 console should be easy. I've done this in other applications, and it was simple.
Instead there was a blank screen and in the JavaScript console:
The message:
Refused to display 'http://localhost:8080/console/header.jsp?jsessionid=2c4c46b7baf3f8f1dfe697fdf382b444' in a frame because it set 'X-Frame-Options' to 'deny'.
Sort of made some sense. After some DuckDuckGo searching I found this referred to an <iframe>
where the frame options didn't allow something or other. Since I didn't have access to the code in question, it wasn't possible to change the tag to to allow the display.
See:
developer.mozilla.org docs HTTP Headers X-Frame-Options
But -- in Spring Boot there is a way to change the behavior. The issue only shows up when Spring Security is enabled, because Spring Security by default configures this option to deny
. But, it's possible to change the configuration.
In a WebSecurityConfigurerAdapter
one must implement a configure(HttpSecurity http)
method, which you're probably already familiar with if you've done much Spring Security. The result will look like this:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.headers().frameOptions().sameOrigin();
http.csrf().ignoringAntMatchers("/console/**");
http.authorizeRequests().antMatchers( ... "/console/**", ...).permitAll();
}
}
The first sets <iframe>
options to SAME ORIGIN
, which is the cure for the above issue.
The other two lines fix other issues that boil down to allowing access to the /console
URL's so that you can view and work with the H2 console.
The contents of this method of course need to be intermingled with your existing HttpSecurity
configuration.
See also:
-
https://stackoverflow.com/questions/28647136
-
stackoverflow.com/questions/27358966
-
stackoverflow.com/questions/20498831