; Date: Wed Sep 06 2017
Tags: Spring Boot »»»»
Error messages coming out of Spring can be more than inscrutable. As discussed in my solution to transient unsaved properties, I'm defining a REST API using Swagger/OpenAPI targeting a Spring Boot implementation. The object models are complex, with lots of nested objects. Today's inscrutable message, "detached entity passed to persist", doesn't make much sense as a phrase does it? Detached? Detached Entity? Say what? Turns out it's not that hard a problem to fix.
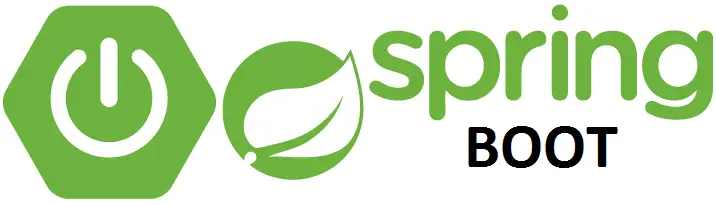
Let's start with the error:
2017-09-06 11:38:02.311 DEBUG 97766 --- [nio-8080-exec-5] o.s.b.w.f.OrderedRequestContextFilter : Cleared thread-bound request context: org.apache.catalina.connector.RequestFacade@73331f72
2017-09-06 11:38:02.315 ERROR 97766 --- [nio-8080-exec-5] o.a.c.c.C.[.[.[/].[dispatcherServlet] : Servlet.service() for servlet [dispatcherServlet] in context with path [] threw exception [Request processing failed; nested exception is org.springframework.dao.InvalidDataAccessApiUsageException: detached entity passed to persist: com.amzur.orangebuttonapi.model.InverterCharacteristics; nested exception is org.hibernate.PersistentObjectException: detached entity passed to persist: com.amzur.orangebuttonapi.model.InverterCharacteristics] with root cause
org.hibernate.PersistentObjectException: detached entity passed to persist: com.amzur.orangebuttonapi.model.InverterCharacteristics
at org.hibernate.event.internal.DefaultPersistEventListener.onPersist(DefaultPersistEventListener.java:124) ~[hibernate-core-5.0.12.Final.jar:5.0.12.Final]
at org.hibernate.internal.SessionImpl.firePersist(SessionImpl.java:765) ~[hibernate-core-5.0.12.Final.jar:5.0.12.Final]
at org.hibernate.internal.SessionImpl.persist(SessionImpl.java:758) ~[hibernate-core-5.0.12.Final.jar:5.0.12.Final]
at org.hibernate.jpa.event.internal.core.JpaPersistEventListener$1.cascade(JpaPersistEventListener.java:80) ~[hibernate-entitymanager-5.0.12.Final.jar:5.0.12.Final]
at org.hibernate.engine.internal.Cascade.cascadeToOne(Cascade.java:398) ~[hibernate-core-5.0.12.Final.jar:5.0.12.Final]
at org.hibernate.engine.internal.Cascade.cascadeAssociation(Cascade.java:323) ~[hibernate-core-5.0.12.Final.jar:5.0.12.Final]
at org.hibernate.engine.internal.Cascade.cascadeProperty(Cascade.java:162) ~[hibernate-core-5.0.12.Final.jar:5.0.12.Final]
at org.hibernate.engine.internal.Cascade.cascadeCollectionElements(Cascade.java:431) ~[hibernate-core-5.0.12.Final.jar:5.0.12.Final]
at org.hibernate.engine.internal.Cascade.cascadeCollection(Cascade.java:363) ~[hibernate-core-5.0.12.Final.jar:5.0.12.Final]
at org.hibernate.engine.internal.Cascade.cascadeAssociation(Cascade.java:326) ~[hibernate-core-5.0.12.Final.jar:5.0.12.Final]
at org.hibernate.engine.internal.Cascade.cascadeProperty(Cascade.java:162) ~[hibernate-core-5.0.12.Final.jar:5.0.12.Final]
at org.hibernate.engine.internal.Cascade.cascadeComponent(Cascade.java:294) ~[hibernate-core-5.0.12.Final.jar:5.0.12.Final]
at org.hibernate.engine.internal.Cascade.cascadeProperty(Cascade.java:177) ~[hibernate-core-5.0.12.Final.jar:5.0.12.Final]
at org.hibernate.engine.internal.Cascade.cascade(Cascade.java:111) ~[hibernate-core-5.0.12.Final.jar:5.0.12.Final]
This stack trace actually goes on for a couple more screen-fulls. That's a sign right there of too much complexity.
The Controller method is what you'd expect -- it handles a POST operation on a given URL, and has a ton of Annotations for this and that purpose:
@ApiOperation(value = "", notes = "Create a new Inverter", response = Inverter.class, tags = {},
produces="application/json, application/xml", consumes="application/json, application/xml")
@ApiResponses(value = { @ApiResponse(code = 200, message = "OK", response = Inverter.class) })
@RequestMapping(value = "/inverters", method = RequestMethod.POST,
consumes = "application/*", produces = "application/json; charset=UTF-8")
ResponseEntity<Inverter> createInverter(
@ApiParam(value = "The Inverter object to create", required = true) @Valid @RequestBody Inverter inverter,
@RequestHeader(value = "Accept", required = false) String accept) throws Exception {
logger.info("CONTROLLER createInverter " + inverter.toString());
return new ResponseEntity<Inverter>(invertersService.createInverter(inverter), HttpStatus.OK);
}
The debugging output includes this message indicating it got into the controller method, and the object was correctly structured:
2017-09-06 11:38:02.145 INFO 97766 --- [nio-8080-exec-5] c.a.o.api.InverterController : CONTROLLER createInverter class Inverter {
details: class DeviceDetails {
costPerUnit: 1230.0
manuals: http://sma.de/product/documentation
manufactureDate: class EpochTime {
secondsSinceEpoch: 434356
}
purchaseDate: class EpochTime {
secondsSinceEpoch: 434356
}
numberOfUnits: 0
warrantyPromise: It works fer real!
}
nameplate: class InverterNameplate {
efficiencyCEC: 90.0
efficiencyEURO: 90.0
maximumEfficiency: 90.0
nightConsumption: 10.0
topology: transformer
characteristics: [class InverterCharacteristics {
maximumInputCurrentPerMPPT: 0.0
maximumPowerDC: 0.0
maximumShortCircuitCurrentPerMPPT: 0.0
maximumVoltageDC: 0.0
mpptVoltageRangeDC: class Range {
start: 0.0
end: 0.0
type: 0.0
}
operatingVoltageRangeDC: class Range {
start: 0.0
end: 0.0
type: 0.0
}
nominalPowerDC: 0.0
numberOfInputsPerMPPT: 0
numberOfMPPT: 0
startupPowerDC: 0.0
startupVoltageDC: 0.0
maximumCurrentAC: 0.0
maximumPowerAC: 0.0
outputFrequencyRange: class Range {
start: 0.0
end: 0.0
type: 0.0
}
outputVoltageRange: class Range {
start: 0.0
end: 0.0
type: 0.0
}
powerFactor: 0.0
ratedFrequency: 0.0
ratedPowerAC: 0.0
ratedVoltageAC: 0.0
disconnectionType: string
}]
}
api: class Communication {
documentation: http://sma.de/product/documentation
type: ModBus
}
measurements: [class Measurement {
}]
firmwareVersion: class Firmware {
com.amzur.orangebuttonapi.model.Firmware@578d9cb9
}
addresses: [class DeviceAddress {
value: 192.168.1.100
type: lan
}]
omActivities: [class OperationsAndMaintenanceActivity {
}]
hasBuiltInMeter: false
inverterType: string
phaseType: single_phase
}
The Service class just takes this and saves it to the Repository, so what's the problem?
The solution to this is pretty simple but we must go over a few things. First, let's return to the definition in Hibernate for entity states ...
Section 5 of the Hibernate User Guide has this helpful explanation:
Persistent data has a state in relation to both a persistence context and the underlying database.
transient the entity has just been instantiated and is not associated with a persistence context. It has no persistent representation in the database and typically no identifier value has been assigned (unless the assigned generator was used).
managed, or persistent the entity has an associated identifier and is associated with a persistence context. It may or may not physically exist in the database yet.
detached the entity has an associated identifier, but is no longer associated with a persistence context (usually because the persistence context was closed or the instance was evicted from the context)
removed the entity has an associated identifier and is associated with a persistence context, however it is scheduled for removal from the database.
Detached means the Entity has an Identifier? What that means is the Entity, the class being persisted, has an @Id annotation on a field, and that this particular instance of the Entity has a value in that field. The problem is that this instance is "not associated with a persistent context" meaning it hasn't been saved to the database.
In this case the issue is an ID field in the JSON object being POST'd. Here's the relevant excerpt:
...
"characteristics": [ {
"disconnectionType": "string",
"inverterCharacteristicsID": 0,
"maximumCurrentAC": 0,
"maximumInputCurrentPerMPPT": 0,
"maximumPowerAC": 0,
...
} ]
...
The inverterCharacteristicsID
field corresponds to this:
@Entity
public class InverterCharacteristics {
@Id @GeneratedValue
@JsonProperty("inverterCharacteristicsID")
private Integer inverterCharacteristicsID;
...
}
The InverterCharacteristics object has @Id and @GeneratedValue annotations. This key is then used as the Primary Key of the generated database table, and Hibernate will automatically generate the ID value for us.
Therefore, when the JSON specifies a value for inverterCharacteristicsID
, that field in the object has a value in it. Because it has a value, it's in this detached state described above. And when the code tries to save the object, Hibernate throws the error because it cannot assign an ID to the object when it already has an ID.
The solution? Delete that field from the JSON.
Oh... why doesn't the inverterCharacteristicsID
get printed in the object dump above? That's pretty simple, the toString
method in question simply doesn't print the value of that field.