Tags: Amazon Web Services »»»» AWS Lambda
AWS Lambda has excellent support for Node.js code. As of this writing Lambda supports Node.js 8.10, meaning we have excellent async function support and other modern ES2015+ language features.
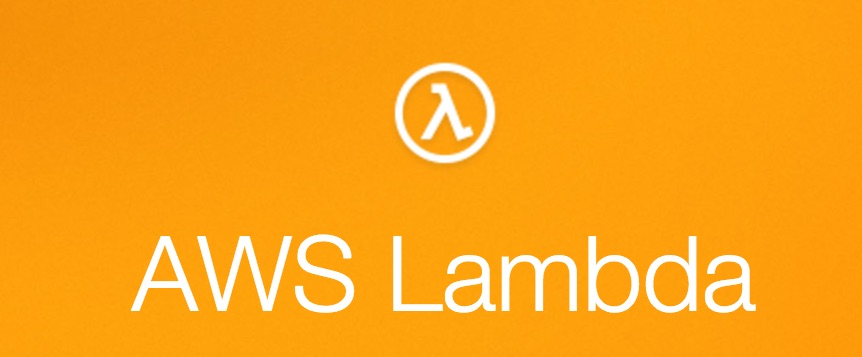
Log-in to your AWS Console and search for AWS Lambda among the many AWS services. You'll end up at
https://console.aws.amazon.com/lambda/home
In the upper toolbar, make sure your region is set as you wish.
Then click on the Create Function button.
Amazon makes available a large set of blueprints and sample applications. But we'll ignore all that, and instead create a blank Node.js application using the Author from Scratch choice.
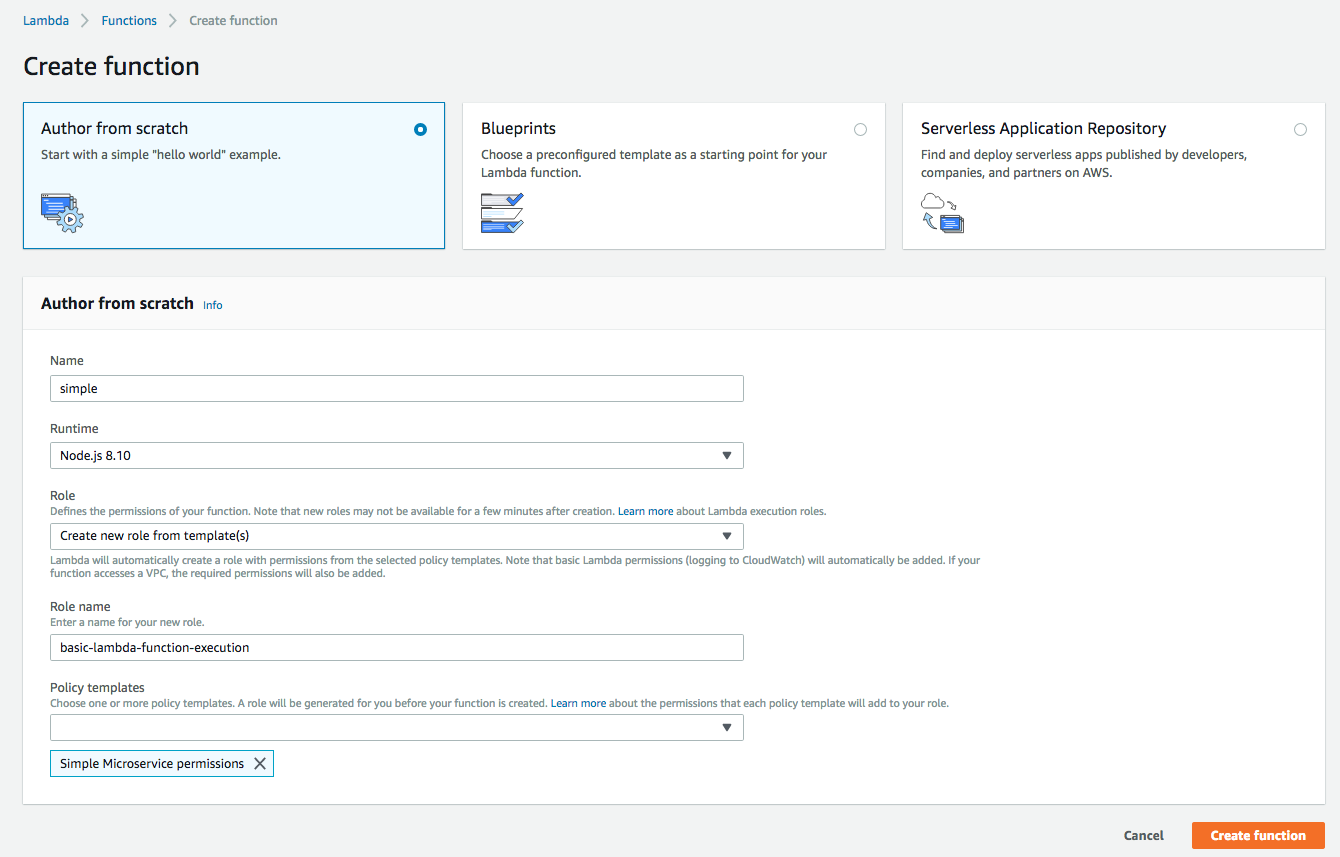
Fill in the form as shown. We have given a function name, and selected Node.js 8.10. Then we create a new Role for this function, giving it "Simple Microservice Permissions".
After clicking on Create Function we end up on the function editor window. There is a lot to this window, and we'll go over the sections at the bottom of this page.
Let's leave the Lambda triggers alone for now, and focus on the code. By default we're given this code in the code editor (see images below):
exports.handler = async (event) => {
// TODO implement
return 'Hello from Lambda!'
};
This is a simple Node.js module containing an async
function. Obviously it will return the given string. Because it is an async function, the return value turns into a Promise, and the AWS Lambda framework will already know how to deal with that Promise.
This is the essence of a Function, in a mathematical sense. In mathematics, a function is a magic box where you drop in data, and out pops a result. Here we have input, the event object, and output, the Promise resulting from executing the function.
Let's go ahead and execute this function to get our feet wet.
At the top of the screen is a row of buttons that includes a Test button. Next to it is a dropdown for Test Conditions which is a fancy phrase for the data that will be sent into the function when we click on the Test button.
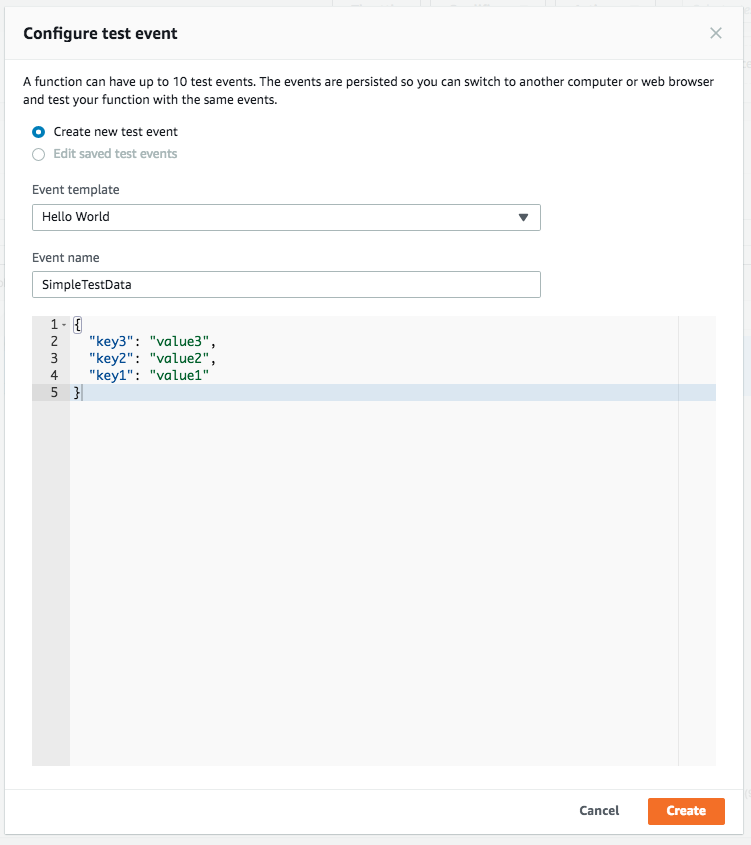
With a Test Condition defined, click on the Test button. This sets up a container to hold the Lambda function, and executes the function using the data in the selected Test Condition.
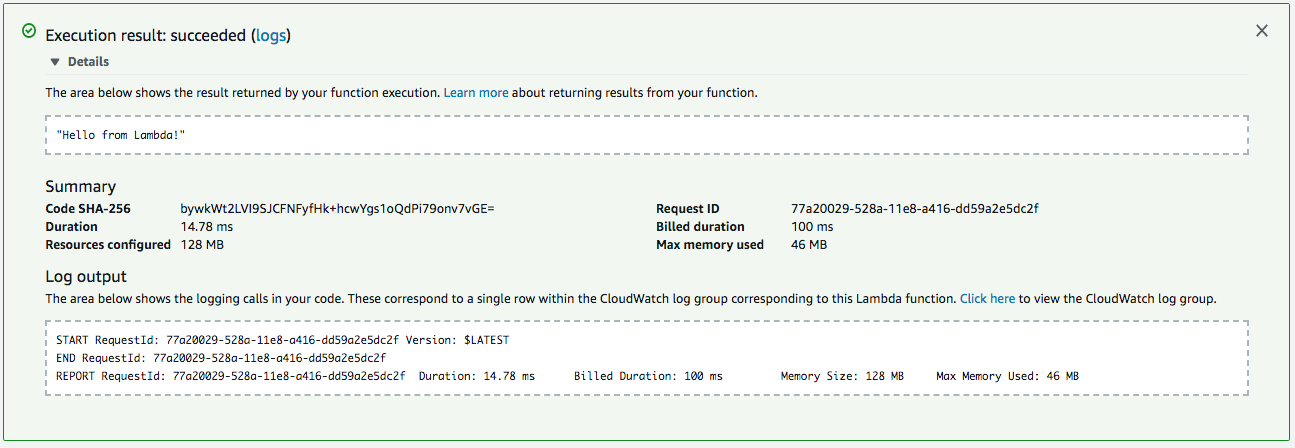
This shows the results of executing the Lambda function. Everything is pretty much self-explanatory, and what we would expect. The output is the string returned by the function, as expected. And we have some reporting output detailing the execution time and resources consumed.
Since this did nothing with the provided test data, let's make a small change so we can inspect the data.
exports.handler = async (event) => {
return event;
};
Re-run the test condition, and we get the following:
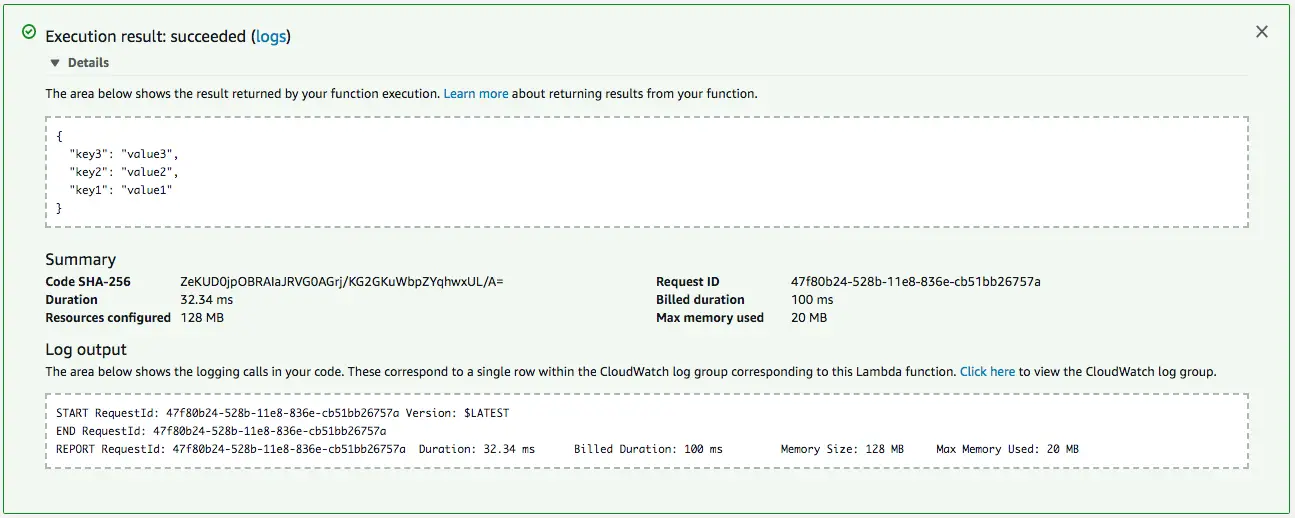
This time around we simply return the event object. Conveniently in the Lambda results log, it pretty-printed the object. The object matches the value we input using the test condition.
Let's try this to make an actual action of some kind:
exports.handler = async (event) => {
return event.a * event.b;
};
We're expecting two numbers in a
and b
fields, and we'll multiply them together and return the result.
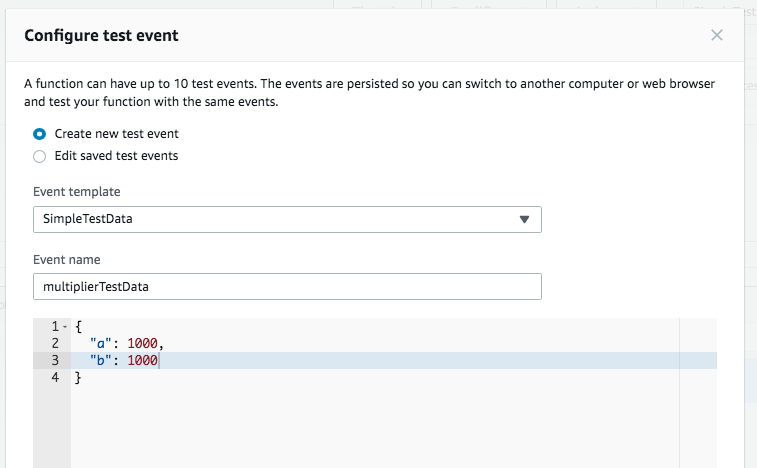
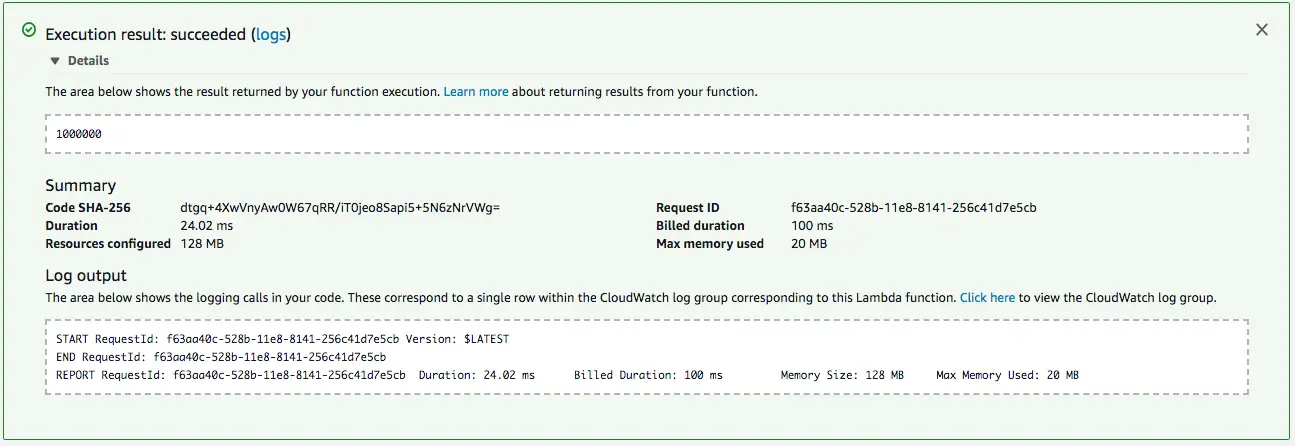
And, indeed, 1000 * 1000 is 1 million.
Lambda Function Editor panes
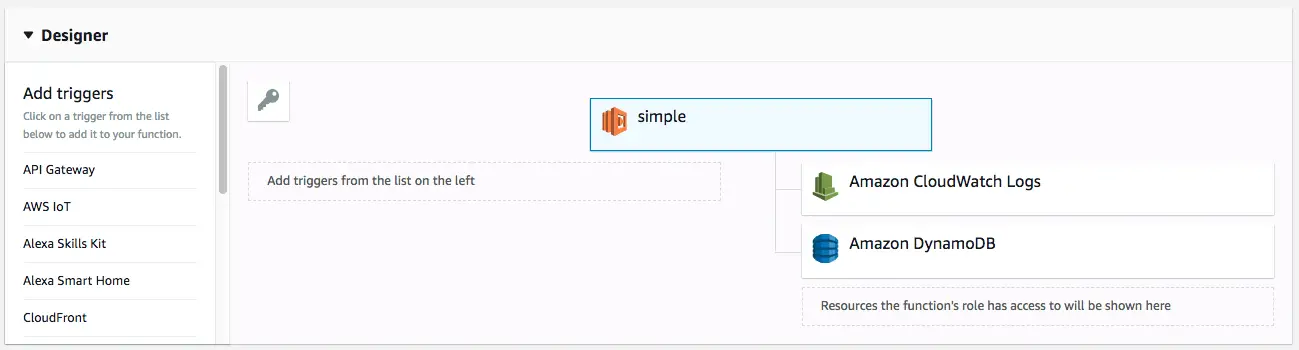
Configuring the ways this Lambda function can be triggered.
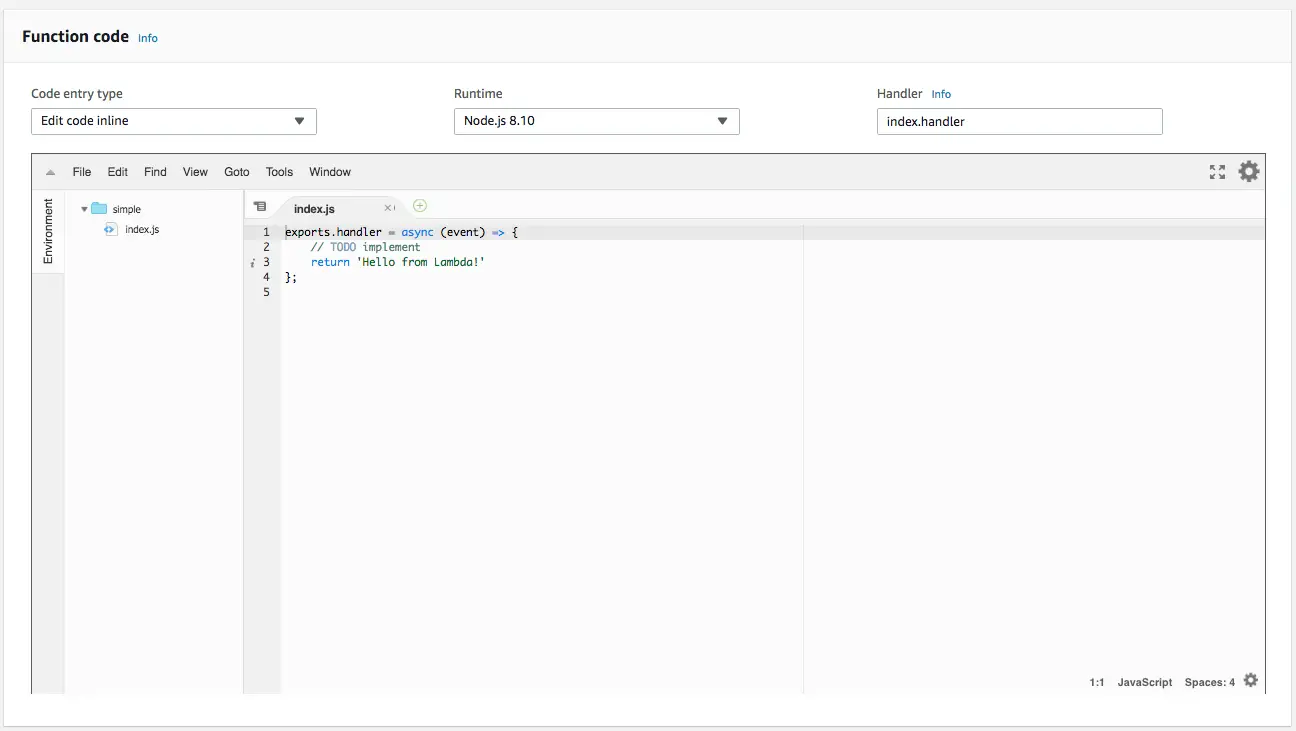
The inline-editor to edit your function code. We can also edit function code on our laptop, and upload the code to the Lambda service.

We can set environment variables in this section - presumably to support injecting configuration data.

Tags help to organize your Lambda functions by groups. Since it's free to create as many Lambda functions as we like, we may end up with hundreds of functions. Therefore it's necessary to sift through the functions as we work. Tags is a simple mechanism for sifting through the list of your Lambda functions.
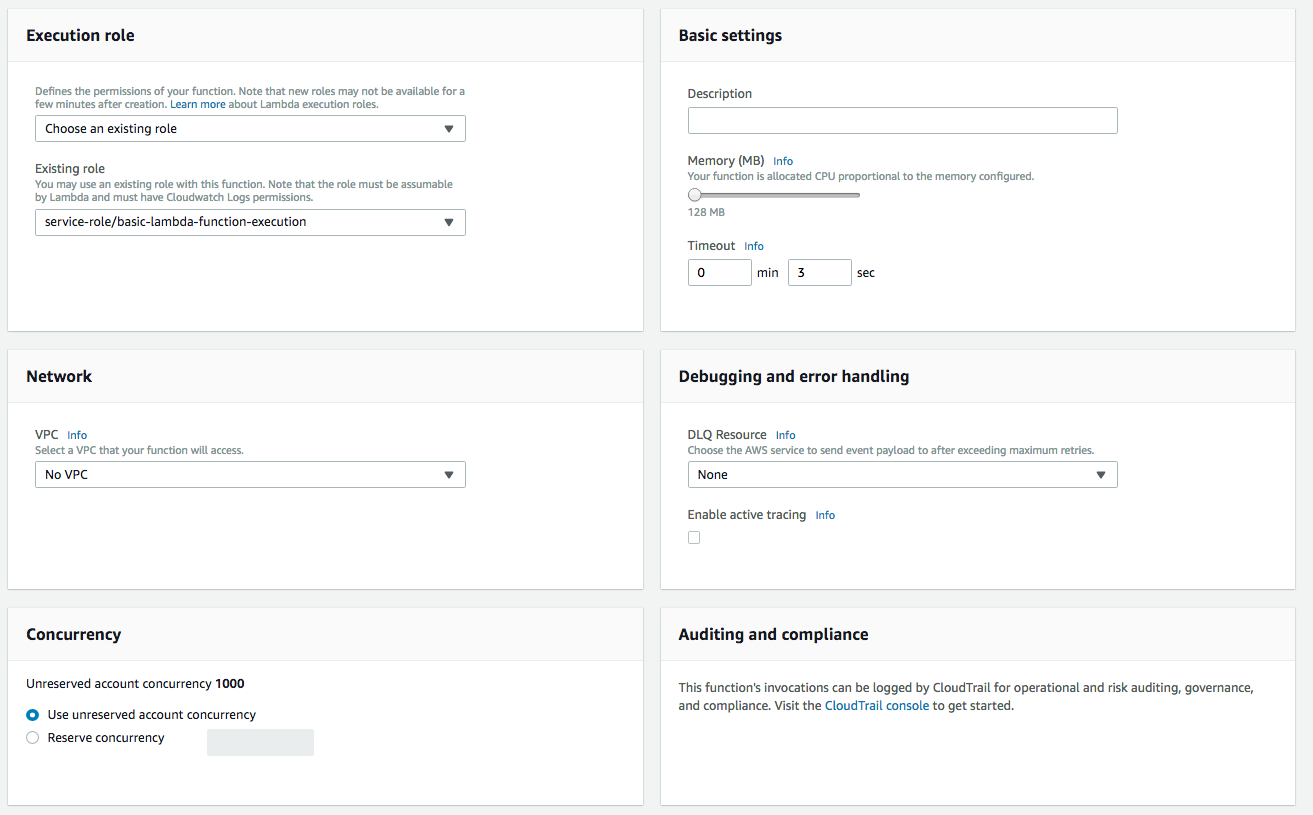