; Date: Sun Feb 25 2018
Tags: Node.JS »»»» JavaScript »»»»
Fast advances in the JavaScript language mean the new features are not evenly distributed. Fortunately the JavaScript language is flexible enough that in many cases new features can be retrofitted by using a polyfill among other techniques. One of those techniques is Babel, a so-called Transpiler that can convert code using new features into equivalent code using old features, so that a programmer can code with the new features and still deploy to older JavaScript implementations.
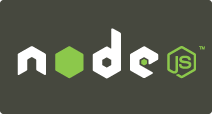
The Babel transpiler (
http://babeljs.io/) is a great way to use cutting edge JavaScript features on older implementations. The word transpile means Babel rewrites JavaScript code into other JavaScript code, specifically to rewrite ES-2015 or ES-2016 features to older JavaScript code. Babel does this by converting JavaScript source to an abstract syntax tree, then manipulating that tree to rewrite the code using older JavaScript features, and then writing that tree to a JavaScript source code file.
Put another way, Babel rewrites JavaScript code into JavaScript code, applying desired transformations such as converting ES2015/2016 features into ES5 code that can run in a web browser.
Many use Babel to experiment with new JavaScript feature proposals working their way through the ECMAscript committee (TC-39). Others use Babel to use new JavaScript features in projects on JavaScript engines that do not support those features.
When should one use Babel?
The general case is: Whenever you're deploying JavaScript code to an older JavaScript platform, while desiring to use the new language features.
There's a number of variants to that general case. The new language features - async functions primarily - are so compelling that all JavaScript programmers should be itching to use them as soon as possible. While many of the new features are simple syntactic sugar that makes JavaScript a little nicer, some (like async functions) are fundamentally important because it opens a whole new way of writing JavaScript code.
Deploying newer-style code to older browsers This is an issue we've had all along, that old browsers exist and our code (whether it's HTML, CSS or JavaScript) has to accommodate the older browsers. Raise your hands if you remember buttons telling you which browsers are best for viewing each given website? And how many websites have you been locked out of due to refusing to use Internet Explorer? And how many insist on pronouncing it Internet Exploder? Okay, I got on a little tangent, but all those questions are forms of the Old Browser problem.
We just said the new JavaScript paradigm is extremely compelling, but your marketing department knows you need to support ES-5 browsers. ES5 doesn't have the new features and does that mean you're stuck unable to use the new features? In a few years the ES5 browsers will all be replaced and then finally your marketing department will finally admit it's safe to use ES2015/2016/2017 features, but by then there will be ES-2021 features that are extremely compelling.
Babel can rewrite most of the features to run on ES5 browsers today. And when ES2021 rolls around, Babel will surely still exist and will be able to rewrite those new features to run on ES2016 browsers.
Deploying newer-style code to older Node.js implementations A variant of the issue is that Node.js hasn't always supported ES2015/2016/2017 features. If your team has declared that Node.js 0.10.x is the standard to which your code must run, then you must use Babel if you're going to use the new features.
There's a feature you can use today on Node.js 9.x -- ES6 Modules -- that aren't supported by any Node.js 8.x or earlier. And to use them on 9.x even requires an option to enable the feature, because it's so experimental.
If your team really is sticking to 0.10.x it's time to have a serious talk with the Architect. These new features are really really really compelling, and Node.js 8.9.x is about 99% compatible with the ES2015/2016/2017 features -- See
http://node.green/
The big missing feature is ES6 modules and that's not compelling enough to jump through hoops just to use them. Async functions ARE compelling enough to go to the effort.
How to use Babel?
Here's a quick example. In a Node.js project directory type these commands:
$ npm install babel-cli --save
$ npm install babel-plugin-transform-es2015-modules-commonjs --save
$ npm install babel-plugin-transform-async-to-generator --save
Then create a file named .babelrc
containing:
{
"plugins": [
"transform-es2015-modules-commonjs",
"transform-async-to-generator"
]
}
THis declares two transformations -- one rewrites ES6 modules to CommonJS/Node.js format -- the other rewrites async functions to generator functions with a helper.
You can get help with Babel: $ ./node_modules/.bin/babel -help
To transpile one file: $ ./node_modules/.bin/babel moduleName.js -o moduleName-babel.js
Babel has commands to transpile a whole directory structure, to watch the files and dynamically retranspile anything which changes, and so on.
A typical pattern is to put ES2015/2016/2017 source files in a directory named src
and use Babel to transpile everything in that directory into lib
. Your system would then be instructed to execute the files in lib
, and it's the lib
files you would distribute.
I think it's feasible to have different transpilations for different targets. Suppose your code is to run in browsers, and you're able to detect the browser features. You could transpile with one set of transpilation plugins for one set of browsers - and use another set of transpilation plugins for other browsers - and then setup the HTML to load the correct transpilation depending on the browser.