Tags: Vue.js »»»» JavaScript
Many kinds of applications show a tree structure of data items, such as files in a file system. Over the last couple weeks I implemented a simple Markdown editor in Vue.js/Electron - and while the sample application did not show a file-system tree a full version of the application would do so. We use this sort of widget all the time in programs and web applications, but do you know how to implement such a widget? Speaking for myself I could probably work it out - it's nested UL and LI elements with some custom styling and whatnot - but I'd rather use a pre-baked tree component for Vue.js instead. The application shown here demonstrates using one such tree component.
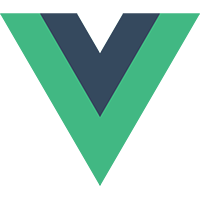
We need the Vue.js CLI tool installed. See Getting started with Vue.js application development
$ vue init webpack-simple sl-vue-tree
If you need a tour of the skeleton see Getting started with Vue.js application development
The sl-tree-vue
widget is the Vue.js tree component we've chosen for this example. The code is available via npm at:
https://www.npmjs.com/package/sl-vue-tree
To help with styling the sl-vue-tree component we'll use the Font Awesome library of icons. It is available via npm at:
https://www.npmjs.com/package/vue-awesome
To install both:
$ cd sl-vue-tree
$ npm install sl-vue-tree vue-awesome --save
$ npm install
In index.html
, make this change:
<div id="example-sl-vue-tree"></div>
In main.js
use this:
import Vue from 'vue'
import App from './App.vue';
import slVueTree from 'sl-vue-tree';
import 'sl-vue-tree/dist/sl-vue-tree-dark.css';
Vue.component('sl-vue-tree', slVueTree);
import 'vue-awesome/icons';
import Icon from 'vue-awesome/components/Icon';
Vue.component('icon', Icon);
new Vue({
el: '#example-sl-vue-tree',
render: h => h(App)
});
The middle section is where we added code, the top and bottom is what the skeleton gave us.
The first section brings in the sl-vue-tree
component. We import the component, then use Vue.component
to register the component globally. We've also imported one of the theme CSS files.
The second section brings in the Font Awesome library. First we bring in the icons, then we bring in the Icon component. With that component we can use one of the icons simply with the <icon>
tag.
Then, change App.vue
to this:
<template>
<div id="app">
<sl-vue-tree v-model="nodes" @nodeclick="nodeClick">
<template slot="toggle" slot-scope="{ node }">
<span v-if="!node.isLeaf">
<icon name="caret-right" v-if="!node.isExpanded"></icon>
<icon name="caret-down" v-if="node.isExpanded"></icon>
</span>
</template>
<template slot="title" slot-scope="{ node }">
<icon name="file" v-if="node.isLeaf"></icon> {{ node.title }} </template>
<template slot="sidebar" slot-scope="{ node }">
<icon name="circle" v-if="node.data.isModified"></icon>
</template>
</sl-vue-tree>
</div>
</template>
<script>
var nodes = [
{title: 'Item1', isLeaf: true},
{title: 'Item2', isLeaf: true, data: { visible: false }},
{title: 'Folder1'},
{
title: 'Folder2', isExpanded: true, children: [
{title: 'Item3', isLeaf: true},
{title: 'Item4', isLeaf: true, data: { isModified: true }}
]
}
];
export default {
name: 'app',
data () {
return {
nodes: nodes
}
},
methods: {
nodeClick(node) {
console.log(node);
}
}
}
</script>
<style>
</style>
We've set up an sl-vue-tree
component, with its data stored in the nodes
object. That object is fairly self-explanatory. It is an array of objects conforming to this declaration:
interface ISlTreeNodeModel<TDataType> {
title: string;
isLeaf?: boolean;
children?: ISlTreeNodeModel<TDataType>[];
isExpanded?: boolean;
isSelected?: boolean;
isDraggable?: boolean;
isSelectable?: boolean;
data?: TDataType; // any serializable user data
}
The only place you're allowed to put custom data is in the data
field. In the example above we've added an isModified
flag to one of items, and then in the sidebar
we use that flag to show an icon.
The interesting thing about this is sl-vue-tree
has several slots into which we can inject our own HTML code. In Vue.js a slot is an injectable part of the Component. Each slot is defined in the component's template, and then in the application's template we can define <template>
elements that are injected into the named slot.
The toggle
slot is applicable only for items with children. It shows a toggle button to control whether the children are visible. The toggle icon is only shown for non-leaf items.
The title
slot renders both an icon to show the item type, as well as the name (the title
field) of the item. The item type icon is only shown for leaf items.
The sidebar
slot appears way over on the right side, and can be used for an extra status indicator. In this case we're planning to show a dot-thing to indicate the file is modified.
The nodeClick
function is, at the moment, solely to show data about each item. Obviously it or any of the other functions supported by this component can be used for actual application-specific purposes.
Once you've made the changes, you can run it on your laptop with: npm run dev
You'll get something like this: