; Date: Fri Jul 20 2018
Tags: Vue.js »»»» JavaScript
Vue.js is an exciting new framework for developing front-end JavaScript applications. It promises to be a "Progressive" web framework, and I don't think they mean in a Political sense but means that Vue.js can be plugged into the part of an application which requires a richer experience. It is also supposed to be Approachable, works directly from HTML, CSS and JavaScript, while being Versatile and Performant. In this example we'll build a starter application, and embed it into an HTML web page. Specifically, this web page.
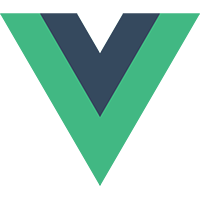
The easiest way to start with Vue.js is to dive right into Single File Templates, and to use the Vue CLI to assist with scaffolding. A Single File Template lets you build a Vue Component all in one file. Don't worry if you don't know yet what a Vue Component is, trust me that it's easier to build them as a single file.
Installing tools
To install Vue CLI - you must first have Node.js installed on your computer. We won't be writing any Node.js code, but the Vue CLI is implemented with Node.js and therefore requires that Node.js is installed on your computer.
If you don't already have Node.js go to
https://nodejs.org/en/ and download the installer shown there.
If you prefer Node.js to be installed using a package manager:
https://nodejs.org/en/download/package-manager/
Once you've done this, type in the command:
$ sudo npm install -g vue-cli
You won't require sudo
on every computer. After running this you have a new command, vue
where the primary purpose is to download application skeletons. The list of official application skeletons is available by typing vue list
. Other skeletons can be downloaded beyond the set listed by that command.
Setup of skeleton example application
For this example we'll simply set up the default application which supports developing applications using Single File Templates. To get started type the command:
$ vue init webpack-simple example-1
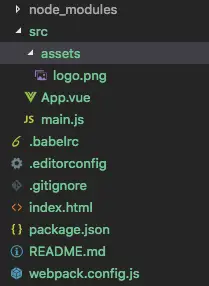
The file App.vue
is the Single File Template which will make up the application. It is easy to create other Vue Components and bring them into such a file.
The file main.js
is the JavaScript executed by running this application.
The file index.html
is the HTML for a container to execute the application.
The file package.json
is required for bringing in packages from the Node.js ecosystem required for building the code. We won't need to touch anything here. It also contains the build process.
The file webpack.config.js
contains the configuration for Webpack that is used in building the code.
Kicking tires
The output instructs you to:
$ cd example-1
$ npm install
$ npm run dev
You'll notice the commands start with npm
. This means we're using npm
to drive the processes, and those processes are defined in package.json
.
After we do this the web browser will automatically open up to this:
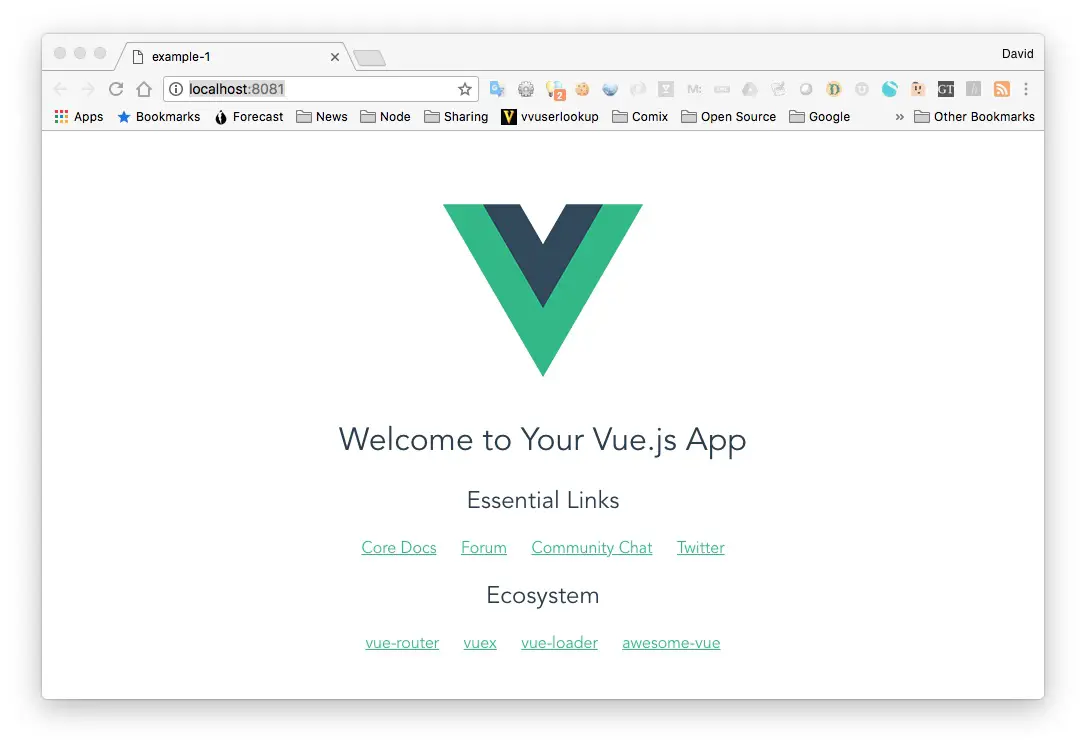
The screen for this is defined in App.vue
. Open that file in your favorite programming editor. We're not going to go too deep into this, fortunately it's fairly straightforward.
The <template>
section describes the HTML of the UI. Comparing it with what's in the browser will be instructive.
The <script>
section contains JavaScript which implements the interactivity. You'll notice in the template a thing - {{ msg }}
- and in the script a section marked data
containing a definition for msg
. This is a core competency of Vue.js, to have data items automatically managed and then appear magically into the template.
The <style>
section contains the CSS for the application.
While you have npm run dev
active you can modify App.vue
and Vue.js will automatically reload it in the browser. Very cool.
Embedding this on a web page.
The next thing we'll do is demonstrate deploying this sample Vue.js app to a website. The app is kind of useless unless it can be used on a website.
In the Vue.js documentation we're told we can put this into an HTML page and the whole world of Vue.js is available:
<script src="https://cdn.jsdelivr.net/npm/vue@2.5.16/dist/vue.js"></script>
That's nice, but we're going to do something different.
In addition to npm run dev
we can use npm run build
to generate a built version of the application.
$ npm run build
> example-1@1.0.0 build /Volumes/Extra/ws/techsparx.com/vue-js-examples/example-1
> cross-env NODE_ENV=production webpack --progress --hide-modules
Hash: 25ea0beedf90a14bba0e
Version: webpack 3.12.0
Time: 14986ms
Asset Size Chunks Chunk Names
logo.png?82b9c7a5a3f405032b1db71a25f67021 6.85 kB [emitted]
build.js 99.2 kB 0 [emitted] main
build.js.map 835 kB 0 [emitted] main
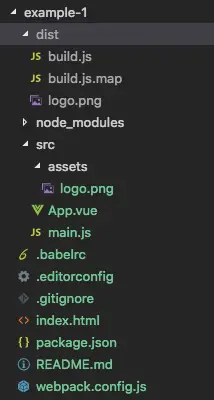
This gives us a few new files with the dist
directory being what's required for deployment. Thanks to everything else it is packaged, minified, and ready to go.
We aren't ready to deploy yet, as we need to do the work to embed this in a web page.
Study index.html
and you'll see it doesn't contain much special. This is where we focus:
<div id="app"></div>
<script src="/dist/build.js"></script>
Then in main.js
we have this:
new Vue({
el: '#app',
render: h => h(App)
})
What happens is that the el:
value is a CSS selector to the DOM location where the application will be mounted. In this example we have a div
with the ID of app
, and as configured Vue.js will attach the application to that div
.
It's rather likely we'll need to attach the application to another location in the DOM - a div
of some other name.
Suppose you're required to mount the application here:
<div id="app-example-01"></div>
Change main.js
to
new Vue({
el: '#app-example-01',
render: h => h(App)
})
And make a corresponding change in index.html
.
The other change that will be required is to modify the script tag. It's very likely you'll be required to deploy the build.js
to a different location than /dist/build.js
.
In webpack.config.js
make this change:
publicPath: '/software-development/vue-js/getting-started/vue-js/',
Then in your deployed HTML page, add this code:
<div id="app-example-01"></div>
<script src="/software-development/vue-js/getting-started/vue-js/build.js"></script>
Then finally in App.vue
change the <script>
tag to this:
<style scoped>
The scoped
keyword performs some CSS magic so that CSS declarations are corralled to affect only the application.
Now you can run npm run build
.
And you'll get something like this:
Links
Vue.js home page:
https://vuejs.org/
Vue.js Guide:
https://vuejs.org/v2/guide/
Vue.js API:
https://vuejs.org/v2/api/
Vue.js examples:
https://vuejs.org/v2/examples/
Vue CLI:
https://www.npmjs.com/package/vue-cli