; Date: Tue Mar 12 2019
Tags: JavaScript »»»» jQuery
Typically with jQuery or Cheerio we read or write strings and numbers to/from the DOM, but what if we must use objects or arrays of data? The jQuery documentation mentions data-* attributes, but the documentation is not entirely clear how to use this for reading/writing objects or arrays to/from the DOM.
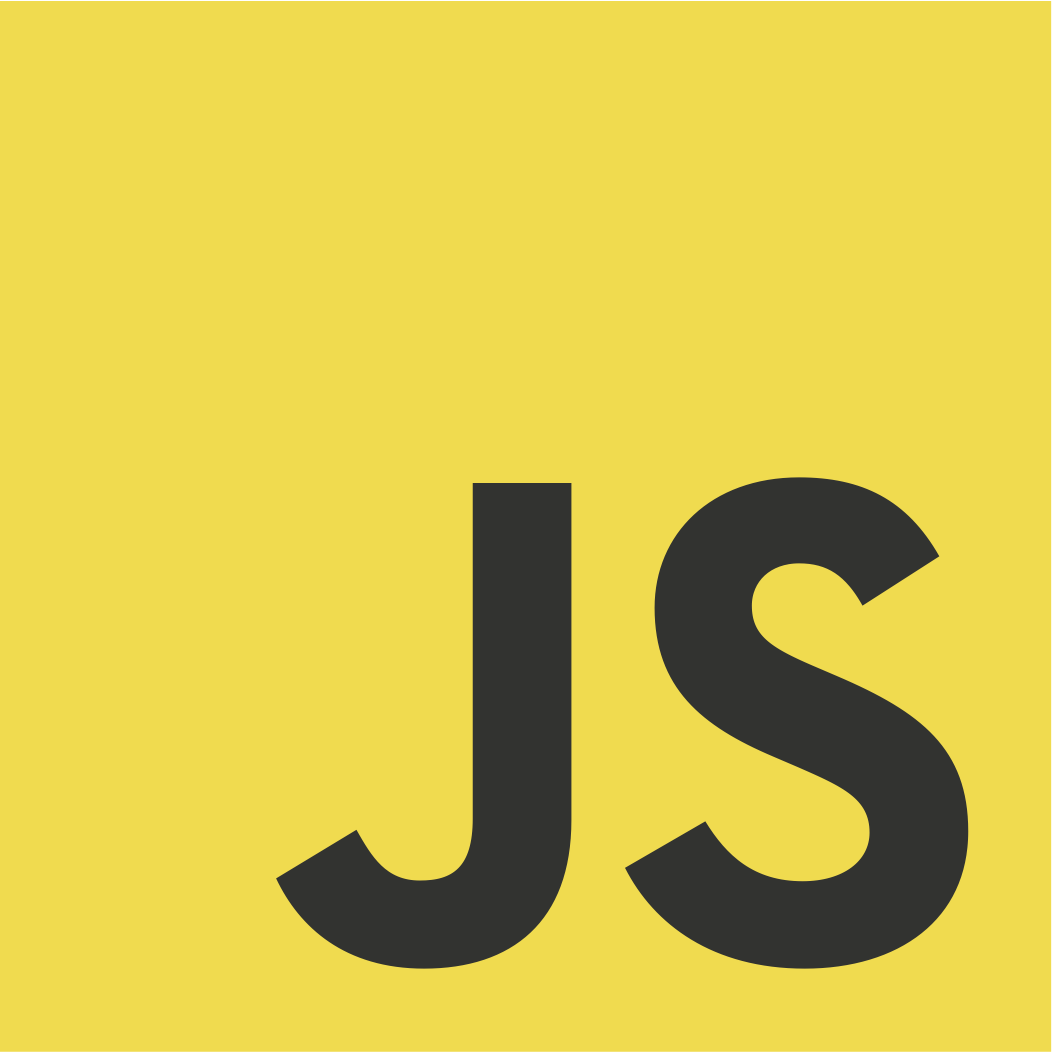
jQuery is a very useful tool to simplify DOM manipulation in browser-side JavaScript, and is useful on the server-side in Node.js using the Cheerio library. The following examples will work in either environment.
The other day I needed to pass an array of strings into a custom HTML element I was defining in AkashaCMS. AkashaCMS uses server-side jQuery-like manipulation implemented with the Cheerio library for modifying HTML files before publishing them to the server. What I thought would work didn't work, and I was about to give up. Instead I went to Codepen and played around using browser-side jQuery to figure it out.
Everything shown below has been verified in Node.js using Cheerio.
Problem statement: How to pass an array of data values using a data-foo=
attribute in an HTML element, such that jQuery recognizes it as an array, and passes a JavaScript Array object to the code.
I tried a number of bad ideas, that I don't remember, so I'll cut to the chase and show the syntax that worked.
<div id="foo"
data-products='[ "camco-14-50-extcord", "conntek-14-50-extcord", "ge-14-50-extcord", "petra-14-50-extcord", "coleman-14-50-extcord", "nucord-14-50-extcord", "utilitech-14-50-extcord" ]'>
</div>
In JavaScript/jQuery this is read as so:
<script>
let d = $("#foo").data('products');
console.log(d);
</script>
Generally speaking the .data
method in jQuery reads/writes data-*
attributes. The data-
part of the name is stripped off, or added, as appropriate, and therefore what the coder sees as a products
attribute is actually data-products
in the DOM.
The key here is using single-quote for the outer level string delimiter, and double-quote to delimit strings within the string. My initial attempt had these reversed, double-quote for the outer delimiter, and single-quote for inner delimiters. That arrangement gave a ton of errors, and using quotes as shown above worked.
To prove this works, I'll embed the example as live code. First we'll show the modifications required to show this visually:
<div id="foo"
data-products='[ "camco-14-50-extcord", "conntek-14-50-extcord", "ge-14-50-extcord", "petra-14-50-extcord", "coleman-14-50-extcord", "nucord-14-50-extcord", "utilitech-14-50-extcord" ]'>
</div>
<ol id="target">
</ol>
<script>
document.addEventListener("DOMContentLoaded", function(){
let d = $("#foo").data('products');
console.log(d);
for (let item of d) {
$("#target").append('<li>'+ item +'</li>');
}
});
</script>
And then we have the live code.
To see the console.log
output you'll need to open the browser console log.
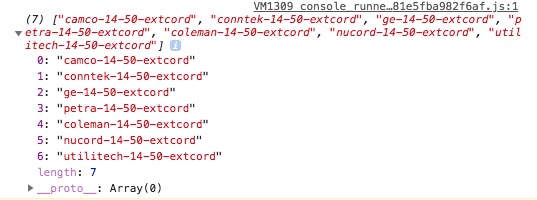
Parsing an array is all well and good, what about an object? The array notation here is the same as JSON, and it appears from the jQuery documentation that a JSON object notation will work, so here goes:
<div id="foo2"
data-products='{ "prod1": "camco-14-50-extcord", "prod2": "conntek-14-50-extcord", "others": [ "ge-14-50-extcord", "petra-14-50-extcord", "coleman-14-50-extcord", "nucord-14-50-extcord", "utilitech-14-50-extcord" ]}'>
</div>
<pre id="target2"></pre>
<script>
document.addEventListener("DOMContentLoaded", function(){
let d = $("#foo2").data('products');
console.log(d);
$("target2").text(JSON.stringify(d));
});
</script>
A few simple modifications to the example. The data is restructured to make an object with three elements, one of which is an array. Instead of interpolating the data item, we'll just stringify it and plop it into a target.
Again, open the console log to see the message.
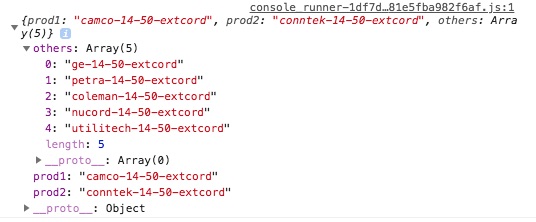